Flask
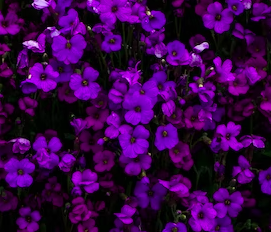
Machine Learning Web App using Flask
In this article, we will develop a machine learning web application using Flask. We will use the Iris dataset. We will load the data, scale it using min-max scaler and split it into train and test set (80-20).
We will use the training set to train the model using Decision Tree Classifier. Then use the test data to calculate the test accuracy, plot confusion matrix and generate classification report. Finally, we will save the model in pickle format.
Code for training and saving the model
import pandas as pd
import numpy as np
import joblib
from sklearn.tree import DecisionTreeClassifier
from sklearn import metrics
df = pd.read_csv("Iris.csv")
#Extracing the features from the dataset
X = df[["SepalLengthCm","SepalWidthCm","PetalLengthCm","PetalWidthCm"]]
#Extract the target from the dataset
y = df["Species"]
#Using the min max scaler
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
#Fitting the data
scaler.fit(X)
# transform data
scaled_X = scaler.transform(X)
#Train test split
from sklearn.model_selection import train_test_split
x_train, x_test, y_train,y_test = train_test_split(scaled_X,y,test_size=0.2,random_state=5)
clf = DecisionTreeClassifier()
clf.fit(x_train,y_train)
#Accuracy
print("Test Accuracy")
y_pred = clf.predict(x_test)
print("Test Accuracy:",metrics.accuracy_score(y_test, y_pred))
#Confusion Matrix
print("Confusion Matrix")
from sklearn.metrics import confusion_matrix
print(confusion_matrix(y_test, y_pred))
#Classification Report
from sklearn.metrics import classification_report
print(classification_report(y_test, y_pred))
joblib.dump(clf,"clf.pkl")
We will make a web application using a Flask and Html. Here we ask user to fill parameters of Iris in an HTML form. The Flask web application will read data from this form after scaling it and feed it to the model. The model will then give us the prediction which will be displayed in the web page.
Code For Flask app
import re
from flask import Flask, request, render_template, flash
import pandas as pd
import joblib
from sklearn.preprocessing import MinMaxScaler
#Declare a Flask app
app = Flask(__name__)
# settings
app.secret_key = "mysecretkey"
@app.route('/',methods=['GET','POST'])
def main():
#If a form is submitted
if request.method == 'POST':
#unpickle classifier
clf = joblib.load('clf.pkl')
#get values through input bars
sepal_length = request.form.get("sepal_length")
print(sepal_length)
sepal_width = request.form.get("sepal_width")
print(sepal_width)
petal_length = request.form.get("petal_length")
print(petal_length)
petal_width = request.form.get("petal_width")
print(petal_width)
#Read orginal data, we need this to normalize the user input before feeding to the model
df = pd.read_csv("Iris.csv")
#Extracing the features from the dataset
X = df[["SepalLengthCm","SepalWidthCm","PetalLengthCm","PetalWidthCm"]]
scaler = MinMaxScaler()
#Fitting the data
scaler.fit(X)
#Put inputs to dataframe
user_X = pd.DataFrame([[sepal_length,sepal_width,petal_length,petal_width]],columns=["SepalLengthCm","SepalWidthCm",'PetalLengthCm','PetalWidthCm'])
print(user_X)
#Transforming the user input
scaled_X = scaler.transform(user_X)
#Get Prediction
prediction = clf.predict(scaled_X)
prediction = prediction[0]
print(prediction)
else:
prediction=""
return render_template("home.html",output=prediction)
#Running the app
if __name__ == '__main__':
app.run(port=5000,debug=True)
Code for Html
<!DOCTYPE html>
<html>
<style>
input[type=number], select {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
input[type=submit] {
width: 100%;
background-color: #4CAF50;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
div {
width: 50%;
margin-left: auto;
margin-right: auto;
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
.header {
padding: 15px;
text-align: center;
background: #1abc9c;
color: white;
font-size: 20px;
}
</style>
<head>
<title>Your Machine Learning App</title>
</head>
<body>
<div class="header">
<h2>Iris Prediction Application</h2>
</div>
<div>
<form name="form", method="POST", style="text-align:center;">
<br>
Sepal Length in cm: <input type="number" step="0.01" name="sepal_length", placeholder="Sepal Length in Cm" required/>
<br><br>
Sepal width in cm: <input type="number" step="0.01" name="sepal_width", placeholder="Sepal Width in Cm" required/>
<br><br>
Petal Length in cm: <input type="number" step="0.01" name="petal_length", placeholder="Petal Length in Cm" required/>
<br><br>
Petal width in cm: <input type="number" step="0.01" name="petal_width", placeholder="Petal Width in Cm" required/>
<br><br>
<button value="Submit">Run</button>
</form>
</div>
<p style="text-align:center;">{{ output }}</p>
</body>
</html>
Output: Landing Page
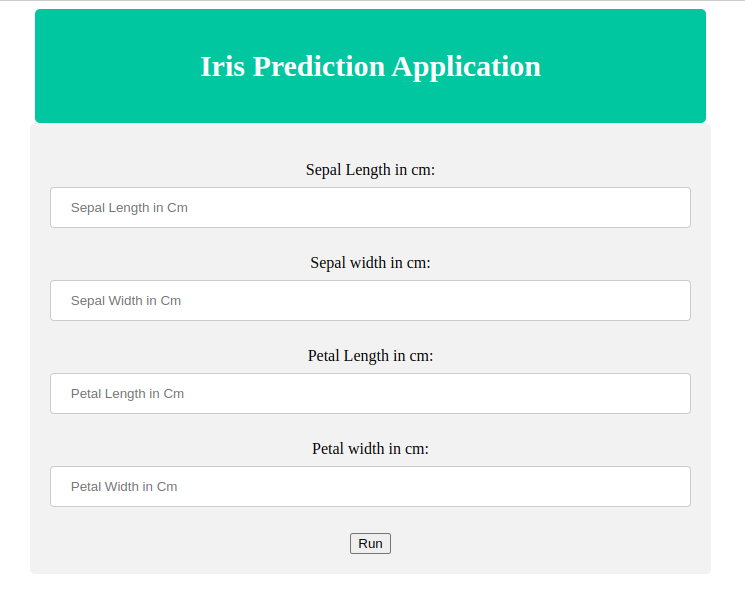
Output: After Entering Data
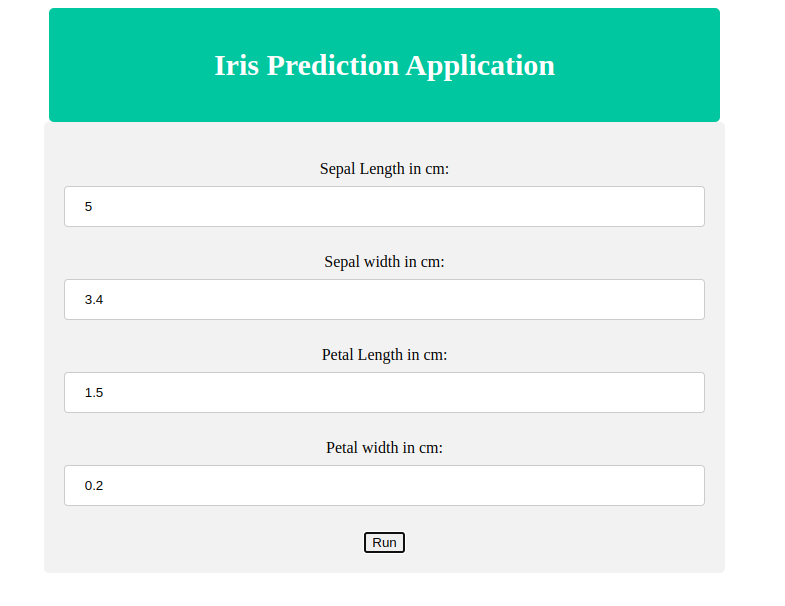
Output: The Prediction
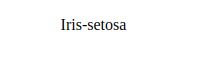
pontu
0
Tags :