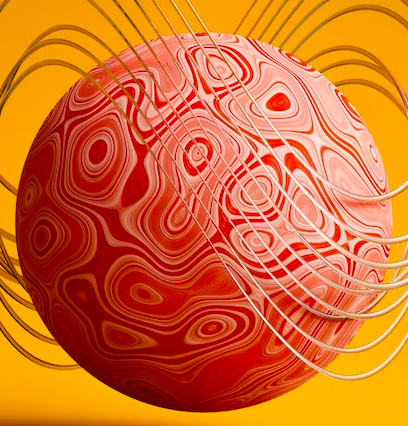
JavaScript Object
They are like a dictionary in Python. This tutorial covers basic of JavaScript objects and how they go together with arrays.
Table of contents:
- Creating Objects
- Updating Objects
- Using variables as key
- Objects in Objects
- Array in Objects
- Objects in Array
- Object in Array in Objects
Creating Objects
The Objects in JavaScript has two parts: keys and values.
In the following example, book is the object. name,pages,Genre etc are the keys while the “Magic Realism” is the value of the key “Genre”.
The value of the key for particular object can be accessed either by object.key or by object[‘key’].
let book = {name: "kafka on the shore",
pages: "505",
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard"
};
console.log(book.year)
console.log(book['pages'])
Output
2002
505
Updating Objects
In the following example, the value of two keys: cover and pages, of the object book has been updated.
let book = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard"
};
console.log(book.cover);
book.cover = 'papaerback'
console.log(book.cover);
book['pages'] = 502;
console.log(book)
Output
hard
papaerback
{ name: ‘kafka on the shore’, pages: 502,Genre: ‘Magic Realism’,Writer: ‘Murakami’,year: ‘2002’,cover: ‘papaerback’
}
Using the Variable as key
We can assign name of key to a variable and use that variable as key.
let book = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard"
};
let author = 'Writer';
console.log(book[author])
Output
Murakami
We can also update the data of the object using the variable as key. In the following example, the variable author is assigned the key ‘writer’. Now, we can update the value of the ‘writer’ using the variable author.
let book = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard"
};
let author = 'Writer';
console.log(book[author])
book[author] = 'Hakuri';
console.log(book[author])
Output
Murakami
Hakuri
Objects in Objects
An object can also be the value of the key of another object. In the following example, we have an object named book. The value of rating and price of this object are themselves an object.
let book = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard",
rating: {
site_1: 5,
site_2: 6,
reader:9,
},
price: {
hardcover:250,
paperpack:150
}
};
console.log(book)
console.log(book.rating)
console.log(book.rating.reader)
console.log(book['price'])
console.log(book['price']['paperpack'])
Output
{name: ‘kafka on the shore’, pages: 505, Genre: ‘Magic Realism’, Writer: ‘Murakami’,year: ‘2002’,cover: ‘hard’,rating: { site_1: 5, site_2: 6, reader: 9 },price: { hardcover: 250, paperpack: 150 }}
{ site_1: 5, site_2: 6, reader: 9 }
9
{ hardcover: 250, paperpack: 150 }
150
Array in Objects
Arrays can also be the values of keys in an object. In the following example, rating and price have array as their values.
let book = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard",
rating: [1,2,3,4],
price: [250,390,630,842]
};
console.log(book.rating)
console.log(book.price[3])
Output
[ 1, 2, 3, 4 ]
842
Objects in Arrays
In the following example, we have an array named Murakami_books, which has two objects as its elements.
let book1 = {name: "kafka on the shore",
pages: 505,
Genre: "Magic Realism",
Writer: "Murakami",
year: "2002",
cover:"hard",
};
let book2 = {name: "1Q84",
pages: 928,
Genre: "Alternate history",
Writer: "Murakami",
year: "2011",
cover:"paperback"
};
Murakami_books = [book1,book2]
console.log(Murakami_books)
Output
[{name: ‘kafka on the shore’, pages: 505, Genre: ‘Magic Realism’,Writer: ‘Murakami’, year: ‘2002’,
cover: ‘hard’ },
{name: ‘1Q84’,pages: 928,Genre: ‘Alternate history’, Writer: ‘Murakami’, year: ‘2011’,cover: ‘paperback’ }
]
Accessing First element of this array
console.log(Murakami_books[0])
Output
{ name: ‘kafka on the shore’,pages: 505,Genre: ‘Magic Realism’, Writer: ‘Murakami’, year: ‘2002’,cover: ‘hard’
}
Accessing the value of ‘Genre’ key of second element of the array.
console.log(Murakami_books[1].Genre)
Output
Alternate history
Objects in array in objects
Objects can have arrays as their key values which have objects as their elements.
Object = {array1: [object1,object2],
array2: [object3,object4]
}
In the following example, book1, book2, book3 and book4 are the objects. array1 contains book1 and book2 as its elements. array2 contains book3 and book4 as its element. ‘Coelho’ is another object, which have keys, set1 and set2. The values of these keys are array1 and array2, respectively.
let book1 = {name:'Alchemist',
pages: 163
};
let book2 = {name:'Zahir',
pages:336
};
array1 = [book1,book2]
let book3 = {name: 'Aleph',
pages:320
};
let book4 = {name: 'Brida',
year: 1990
};
array2 = [book3,book4]
let Coelho = {set1: array1,
set2:array2
};
console.log(Coelho)
Output
{
set1: [ { name: ‘Alchemist’, pages: 163 }, { name: ‘Zahir’, pages: 336 } ],
set2: [ { name: ‘Aleph’, pages: 320 }, { name: ‘Brida’, year: 1990 } ]
}
Accessing Key values
console.log(Coelho.set2[1].year)
Output
1990
Editing the value of the object
Coelho.set2[1]['pages'] = 555
console.log(Coelho.set2[1].pages)
Output
555