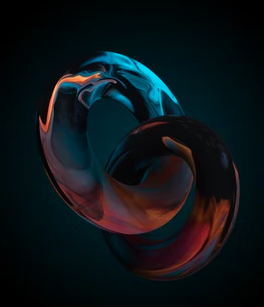
JavaScript Loops
Table of Contents
- While Loops
- Fibonacci sequence using While loops
- Do While Loops
- For Loops
- Nested Loops
- Looping through array
- For of loop
- For in Loop
- Looping over an object
Whlie Loops
While loops allows code to be executed repeatedly based on the given Boolean condition.
while(condition){
//code gets executed as long as the condition is true
}
In the following code the initial value of i is 0. The loop continues to prints the value of i and increase the value of i, till the value of i reaches to 10.
let i = 0;
while(i<10){
console.log(i);
i++;
}
Output
0 1 2 3 4 5 6 7 8 9
We can have a while loop that looks for a value in an array, like this:
The following code searches for the word ‘Zahir’ in the array books. The variable notFound is set to trure. While notFound is true and books.length is greater than zero the code inside the body of while loop keeps on executing. Inside the body of while loop, the first element of the array is compared to the word ‘Zahir’. If this condition is true, the code prints ‘Found my book’ and set notFound to false. If the first element in the array is not ‘Zahir’, the code will print ‘Searching …’ and shifts the elements of books to left, which result in deleting the first element of the array and decreasing index of remaining array by one.
let books = ['Alchemist','Brida','Zahir','Aleph'];
let notFound = true;
while(notFound && books.length > 0){
if(books[0] == 'Zahir'){
console.log('Found my book');
notFound = false;
}
else {
console.log('Searching ... ')
books.shift();
}
}
Output
Searching …
Searching …
Found my book
Fibonacci sequence using While loops
let num1 = 0;
let num2 = 1;
let temp;
fibo_Array = [];
while (fibo_Array.length < 25) {
fibo_Array.push(num1);
temp = num1+num2;
num1=num2;
num2=temp;
}
console.log(fibo_Array);
Output
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368 ]
Do while loop
do {
//code to be executed if the condition is true
}while (condition);
let number;
do {
number = prompt("Please enter a number between 0 and 10");
}while (!(number >=0 && number < 10));
Output
Please enter a number between 0 and 10> 99
Please enter a number between 0 and 10> 5
For Loops
for(initializevariable;condition;statement){
//code to be executed
}
for(let i=0; i<10; i++){
console.log(i)
}
Output
0 1 2 3 4 5 6 7 8 9
We can also use for loop to create a sequence and add values to an array
let array = []
for(let i=0;i<10;i++){
array.push(i);
}
console.log(array)
Output
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Nested Loops
We can run one loop inside another.
In the following example, the outer loop creates and an empty array which is element of another array. This empty array created by the outer loop gets filled with numbers by the inner array.
let array = []
for (let i=0; i<3; i++){
array.push([]);
for(let j=0; j<7; j++){
array[i].push(j);
}
}
console.log(array)
Output
[ [0, 1, 2, 3, 4, 5, 6 ],[ 0, 1, 2, 3,4, 5, 6 ],[0, 1, 2, 3,4, 5, 6 ]]
Looping through array
let array = [some array]
for (initialize variable; variable smaller than arr.length; statement){
//code to be executed
}
let books = ['Alchemist','Aleph','Brida','Zahir']
for (let i=0; i< books.length; i++){
console.log(books[i])
}
Output
Alchemist
Aleph
Brida
Zahir
In the following example, we start by creating an array named books with 7 elements. Inside the for loop we initialize a variable i, check the if value of i is less than length of books array and then increase the value of i by one.
Inside the body we push “…” in the array and print the array itself.
Here as we push new values to an array, the size of the array increases and the condition “i<books.length” is always true. This results in creation of an infinite array.
let books = ['Alchemist','Aleph','Brida','Zahir','Sheep','Airport','Hotel']
for (let i=0;i<books.length;i++){
books.push("...")
console.log(books)
}
For of Loop
An easier way to iterate through array using for loop.
let array = [];
for (let variableName of arr){
//code to be executed
//value of variableName gets updated every iteration
//all values of the array will be variableName once
}
let books = ['Alchemist','Aleph','Brida','Zahir','Sheep','Airport','Hotel']
for (let book of books){
console.log(book);
}
Output
Alchemist
Aleph
Brida
Zahir
Sheep
Airport
Hotel
for in loop
Easier way to navigate through an object.
Following code loops through keys of object book. Inside the body, these keys are passed to the objects and values are printed.
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
for(let key in book){
console.log(book[key])
}
Output
The wild sheep chase
Murakami
1989
3.9
Magic realism
299
The following code also iterate through keys of object and prints them.
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
for(let key in book){
console.log(key)
}
Output
name
Author
Year
Rating
Genre
Pages
Looping Over objects by converting to an array
In the following code we make a array with keys of books, then loop through elements of that array
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
let arrayKeys = Object.keys(book);
for(let key of arrayKeys){
console.log(key)
}
Output
name
Author
Year
Rating
Genre
Pages
Same as above but creating array in initialization part of for loop.
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
for(let key of Object.keys(book)){
console.log(key)
}
Output
name
Author
Year
Rating
Genre
Pages
Creating array of values inside initialization part of for loop.
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
for(let value of Object.values(book)){
console.log(value)
}
Output
The wild sheep chase
Murakami
1989
3.9
Magic realism
299
The following code makes arrays of key value pair and iterates through it.
let book = {
name: "The wild sheep chase",
Author: "Murakami",
Year: '1989',
Rating: '3.9',
Genre:'Magic realism',
Pages: 299
}
for(const [key,value] of Object.entries(book)){
console.log(key,":",value);
}
Output
name : The wild sheep chase
Author : Murakami
Year : 1989
Rating : 3.9
Genre : Magic realism
Pages : 299