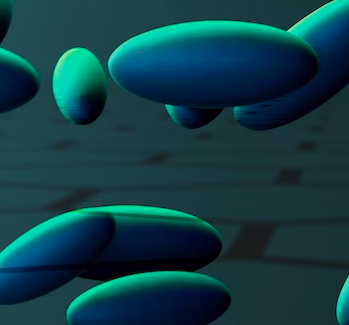
JavaScript Logic Statements
Table of contents
if else
if *some condition is true*,
then,
*a certain action will happen*
else
*another action will happen*
In the following example, user is asked to enter his/her name.
If the name of user is ‘Adele’ the program prints ‘Adele’ otherwise the program prints ‘Bye’.
var name = prompt('Enter your name')
if (name == 'Adele'){
console.log('Hello');
}
else {
console.log('Bye');
}
Output
Enter your name> Antony
Bye
if, else if and else
In the following example the user is asked to enter his/her name.
If the name of user is ‘Antony’, the program prints ‘Welcome Antony’.
If the name of user is ‘Martial’, the program prints ‘Hello Martial’.
When the name of the user is something other than, ‘Antony’ or ‘Martial’. The program prints ‘Bye Bye’.
var name = prompt('Enter your name');
if (name == 'Antony'){
console.log('Welcome: ',name);
}
else if (name == 'Martial'){
console.log('Hello: ',name);
}
else {
console.log('Bye bye');
}
Output
Enter your name> jjj
Bye bye
Output II
Enter your name> Martial
Hello: Martial
Output III
Enter your name> Antony
Welcome: Antony
Conditional ternary operators
operand1 ? operand2 : operand3;
operand1 is the expression that is to be evaluated. If the value of the expression is
true, operand2 gets executed. If the value of the expression is false, operand3 gets
executed.
In the following example, the user is asked to enter his/her name and age. If the entered age is less than 18 the the variable access = ‘denied’ else the variable access=’allowed’
var name = prompt('Enter your name')
var age = prompt('Enter your age');
let access = age < 18 ? "denied" : "allowed"
console.log('You are ',access,name);
Output I
Enter your name> Arpita
Enter your age> 22
You are allowed Arpita
Output II
Enter your name> elon
Enter your age> 13
You are denied elon
Switch
switch (expression) {
case value1:
//code to be executed
break;
case value2:
//code to be executed
break;
case value-n;
//code to be executed
break;
}
In the following example the user is asked to enter the age. Depending upon the value of age the program prints various sentences.
var age = prompt('Enter your age');
switch(age){
case "1":
console.log('Hello baby');
break;
case "2":
console.log('Say Dada');
break;
case "18":
console.log('You are adult now');
break;
}
Output I
Enter your age> 2
Say Dada
Output II
Enter your age> 18
You are adult now
The above example does nothing when the age of user is anything other than 1,2 and 18. By adding default case we can improve our program.
var age = prompt('Enter your age');
switch(age){
case "1":
console.log('Hello baby');
break;
case "2":
console.log('Say Dada');
break;
case "18":
console.log('You are adult now');
break;
default:
console.log('You are not interesting');
break;
}
Output I
Enter your age> 1
Hello baby
Output II
Enter your age> 55
You are not interesting
We can combine multiple cases.
In the following program if the entered grade of student is either F or D. The program prints ‘You failed’.
If the entered grade is either ‘C’ or ‘B’, the program will print ‘You have passed’.
If the entered grade is ‘A’, the program will print ‘Good grades’.
If the entered grade is anything other than, ‘A’, ‘B’, ‘C’, ‘D’ and ‘F’, the program will print ‘I do not know this grade’.
var grade = prompt('Enter your grade');
switch(grade){
case "F":
case "D":
console.log('You failed');
break;
case "C":
case "B":
console.log('You have passed');
break;
case "A":
console.log('Good grades');
break;
default:
console.log('I do not know this grade');
break;
}
Output I
Enter your grade> A
Good grades
Output II
Enter your grade> C
You have passed
Output III
Enter your grade> T
I do not know this grade