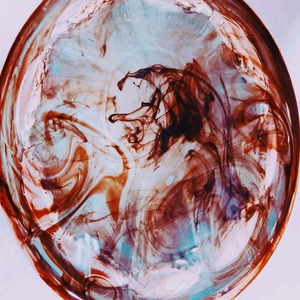
JavaScript function
A function is a block of code that performs a task. It can be called and reused multiple times. You can pass information to a function and it can send information back.
Table of contents
- Basic of function
- A random array function
- Function taking parameters
- Function returning values
- Function with default parameters
- Arrow functions
- Spread operator
- Returning values with arrow function
- Variable scope in function
- Immediately Invoked Function Expression
- Recursive Function
- Nested Function
- Anonymous Function
- Function Callbacks
Basic of function
//Defining function
function hello(){
let name = prompt("What is your name?")
console.log('Hello ',name)
}
//Calling function
hello()
Output
What is your name?> Antony
Hello Antony
A random array function
When we call the function random_array(), it generates a number between 0-5 randomly.
books = ['Antony','Kitchen','Yellow','Culture','Ground','System']
function random_array(){
let x = Math.floor((Math.random() * 5));
console.log(x);
console.log(books[x]);
}
random_array()
Output I
0
Antony
Output II
4
Ground
Function taking parameters
The following function takes two numbers and prints their sum.
function adder(num1,num2){
console.log(num1+num2);
}
adder(2,5)
Output
7
Function returning values
The following function returns the sum of two numbers.
function adder(num1,num2){
let output = num1+num2;
return output;
}
let x = adder(-22,55);
console.log(x);
Output
33
Default Parameters
The following function also sums two numbers and prints their sum. But it also works when the number of arguments passed to the function is not equal to 2.
function adder(num1=0,num2=0){
let output = num1+num2;
console.log('Sum of ',num1,' and ',num2,' is ',output);
}
adder(-22,55)
adder()
adder(22)
adder(1,2,6)
Output
Sum of -22 and 55 is 33
Sum of 0 and 0 is 0
Sum of 22 and 0 is 22
Sum of 1 and 2 is 3
Arrow function
(param1,param2) => body of the function;
Or for no parameters:
() => body of the function
Or for one parameter (no parentheses are needed here);
Param => body of the function
Or for a multiline function with two parameters
(param1,param2) => {
//line1;
//any number of lines;
};
let doingArrowStuff = x => console.log(x);
doingArrowStuff('Cough')
let addingArrow = (num1,num2) => num1+num2;
let sum = addingArrow(1,2);
console.log(sum)
let numSquare = number => number*number;
let square = numSquare(5);
console.log(square)
const arr = ['key','guitar','string'];
arr.forEach(e => console.log(e));
In last example of the arrow function, we have combined the arrow function with certain built-in methods.
We have used foreach() method on an array.
This method executes a certain function for every element in the array.
Output
Cough
3
25
key
guitar
string
Spread Operator
The spread operator is a special operator. It consists of three dots used before a referenced expression or string, and it spreads out the arguments or elements of an array.
let spread = ['cpu','register','counter'];
let computer = ['monitor','board',...spread,'power'];
console.log(computer)
Output
[ ‘monitor’, ‘board’, ‘cpu’, ‘register’, ‘counter’, ‘power’ ]
In the following example, we have a function which takes four arguments and prints their sum. We also have two arrays each having two elements. We pass these arrays to the function using spread operator, which is equivalent to passing four arguments to function.
let array1=[5,6]
let array2=[6,7]
function addFourNumbers(a,b,c,d){
console.log(a+b+c+d);
}
addFourNumbers(...array1,...array2)
Output
24
Returning with Arrow Functions
let addTwoNumbers = (x,y) => {
return x+y;
}
console.log(addTwoNumbers(5,6))
Output
11
Variable scope in functions
Local Variables in functions
function localVar(x){
console.log(x);
}
localVar('Print this');
console.log(x);
Output
Print this
ReferenceError: x is not defined
Here since ‘x’ is part of the function, it cannot be accessed directly outside the function.
Difference between var and let
function testScope(){
if(true){
var x='var';
let y ='let';
console.log(x);
console.log(y);
}
console.log(x);
console.log(y);
}
testScope()
Output
var
let
var
ReferenceError: y is not defined
‘var’ has global scope, while ‘let’ has local scope
const scope
‘const’ has same scope as ‘let’
function constScope(){
const x = 'constatnt1';
if(true){
const y = 'constant2';
console.log(x);
console.log(y);
}
console.log(x);
console.log(y);
}
constScope()
Output
constatnt1
constant2
constatnt1
ReferenceError: y is not defined
Global Variables
Here, ‘x’ is defined outside the function or any block so its scope is global, while ‘y’ which is defined inside the function with ‘let’ has limited scope.
x = 'i am global';
function scope(){
let y = 'i am local';
if(true){
console.log(x);
console.log(y);
}
}
scope();
console.log(x);
console.log(y);
Output
i am global
i am local
i am global
ReferenceError: y is not defined
Immediately invoked function expression
The immediately invoked function expression (IIFE) is a way of expressing a function so that it gets invoked immediately. It is anonymous, it does not have a name and it is self-executing. This can be useful when you want to initialize something using this function. It is also used in many design patterns, for example, to create private and public variables and functions.
(function(){
console.log("Hello I am immediately invoked");
})();
(function(a,b){
console.log(a,b);
})(5,7);
(()=>{
console.log("run right away");
})();
Output
Hello I am immediately invoked
5 7
run right away
Recursive Function
We can call a function inside the body of same function.
function getRecursive(num){
console.log(num);
if(num>0){
getRecursive(--num);
}
}
getRecursive(5);
Output
5
4
3
2
1
0
Power recursive function
function getPower(num,power){
if(power == 0){
return 1;
}
return (num*getPower(num,power-1))
}
let output = getPower(5,5);
console.log(output)
Output
3125
Nested Functions
We can have functions inside functions.
function outerFunction(number){
console.log('the number is: ',number);
innerFunction(number);
function innerFunction(number){
console.log('Square of number ',number,'is ',number*number);
}
}
Output
the number is: 5
Square of number 5 is 25
//trying to accessing inner function
function outerFunction(number){
console.log('the number is: ',number);
innerFunction(number);
function innerFunction(number){
console.log('Square of number ',number,'is ',number*number);
}
}
innerFunction(5)
Output
ReferenceError: innerFunction is not defined
Anonymous Functions
We can also create functions without names if we store them inside variables. We call these functions anonymous.
let functionVar = function(){
console.log('This is variable of anonymous function')
};
functionVar();
Output
This is variable of anonymous function
Example 2
let functionVar = function(number){
console.log('This is variable of anonymous function')
console.log('the number is: ',number);
};
functionVar(3);
Output
This is variable of anonymous function
the number is: 3
Function Callbacks
Here is an example of passing a function as an argument to another function. functionOne and number are the arguments to the functionTwo.
let functionOne = function(number){
console.log('This is functionOne');
let output = number*number*number;
console.log('the number is: ',output);
return output;
};
function functionTwo(functionOne,number){
console.log('This is functionTwo');
let x = functionOne(number);
let output = x+3;
console.log(output);
}
functionTwo(functionOne,2)
Output
This is functionTwo
This is functionOne
the number is: 8
11