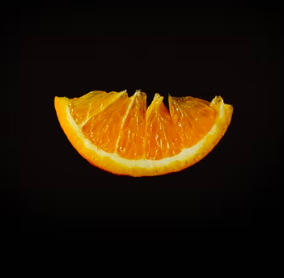
JavaScript break and continue
Table of Contents
break will stop the loop and move on to the code below the loop. continue will stop the current iteration and move back to the top of the loop, checking the condition (or in the case of a for loop, performing the statement and then checking the condition).
break
In the following code, we have an array containing four objects. The for loop iterate through this array and looks for the object whose value for key name is ‘Chocolat’. After encountering this value. The code will print values of name and author of this object and stops looking further in that array i.e break the loop.
novels = [{name:'Wild sheep chase',
Year:1982,
Rating:3.9,
Author:'Murakami'},
{name:'Kafka on the shore',
Year:2002,
Rating:4.1,
Author:'Murakami'},
{name:'Chocolat',
Year:1999,
Rating:4,
Author:'Harris'
},
{name:'Before the Coffee Gets Cold',
Year:2015,
Rating:3.8,
Author:'Kawaguchi'
}
]
for(let novel of novels){
if(novel.name == 'Chocolat'){
console.log(novel.name," is written by ",novel.Author);
break;
}
console.log('Searching for Chocolat')
}
Output
Searching for Chocolat
Searching for Chocolat
Chocolat is written by Harris
continue
The following code looks for the books written by ‘Murakami’. After encountering every object (book) which has value of key author as ‘Murakami’ its increase the count by one and does not run the portion of the loop below continue.
novels = [{name:'Wild sheep chase',
Year:1982,
Rating:3.9,
Author:'Murakami'},
{name:'Before the Coffee Gets Cold',
Year:2015,
Rating:3.8,
Author:'Kawaguchi'
},
{name:'Chocolat',
Year:1999,
Rating:4,
Author:'Harris'
},
{name:'Kafka on the shore',
Year:2002,
Rating:4.1,
Author:'Murakami'},
]
let count = 0;
for(let novel of novels){
if(novel.Author == 'Murakami'){
console.log(novel.name," is written by ",novel.Author);
count += 1;
continue;
}
console.log('Searching for novels by Murakami')
}
console.log('Total novels by Murakami: ',count);
Output
Wild sheep chase is written by Murakami
Searching for novels by Murakami
Searching for novels by Murakami
Kafka on the shore is written by Murakami
Total novels by Murakami: 2
Break and labeled blocks
Here, we have four arrays, the array ‘authors’ has other three arrays as its element.
We have two labels ‘outer’ and ‘inner’. The outer loop iterates through the ‘authors’ array and inner loop iterates through the arrays which are element of ‘authors’ array. The inner loop searches for word ‘Kitchen’ in each inner array and breaks the inner loop after encountering one.
author1 = ['Alchemist','Brida','Zahir']
author2 = ['Airport','Hotel']
author3 = ['Kitchen','Tugumi']
authors = [author1,author2,author3]
outer:
for(let author of authors){
inner:
for(let book of author){
if(book == 'Kitchen'){
console.log(book);
break inner;
}
}
console.log('Searching for kitchen...');
}
Outer
Searching for kitchen…
Searching for kitchen…
Kitchen
Searching for kitchen…
This code is similar to the code above except for the fact that it breaks the outer loop after encountering the desired keyword.
author1 = ['Alchemist','Brida','Zahir']
author2 = ['Airport','Hotel']
author3 = ['Kitchen','Tugumi']
authors = [author1,author2,author3]
outer:
for(let author of authors){
inner:
for(let book of author){
if(book == 'Kitchen'){
console.log(book);
break outer;
}
}
console.log('Searching for kitchen...');
}
Output
Searching for kitchen…
Searching for kitchen…
Kitchen