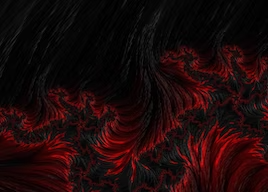
Hello Django
The following tutorial has been operated on Ubuntu 22.04.02 with the Python Version 3.10.6
Create a virtual Environment
mkdir Django_Lessons
cd Django_Lessons
python3 -m venv venv
source venv/bin/activate
Install Django
pip3 install django==4.1.7
Output
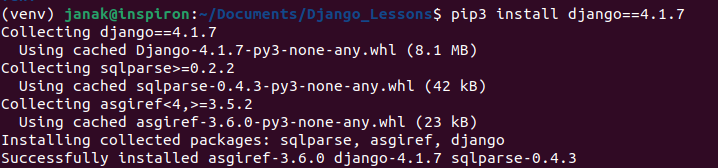
Start a Django Projects
#django-admin startproject django_project
django-admin startproject django_lessons
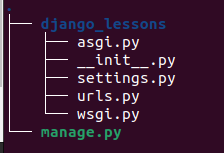
- __init__.py indicates that the files in the folder are part of a Python package. Without this file, we cannot import files from another directory.
- asgi.py allows for an optional Asynchronous Server Gateway Interface to be run.
- settings.py controls our Django project’s overall settings
- urls.py tell Django which pages to build in response to a browser or URL request
- wsgi.py stands for Web Server Gateway Interface which helps Django serve our eventual web pages.
Running Django
python3 manage.py runserver
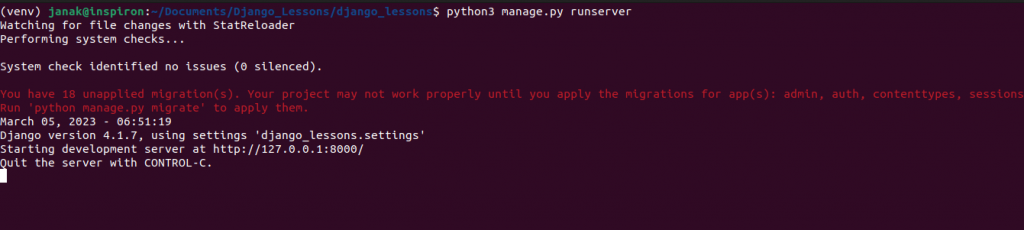
Note that the full command line output will contain additional information including a warning about 18 unapplied migrations. Technically, this warning doesn’t matter at this point. Django is complaining that we have not yet “migrated” our initial database. Since, warnings are annoying we can remove it with following code.
python3 manage.py migrate
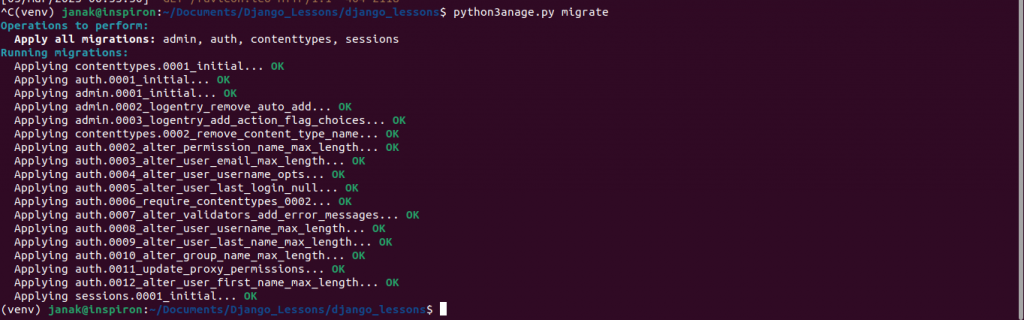
Django created a SQLite database and migrated its built-in apps provided for us. This is represented by the new file db.sqlite3 in our directory.
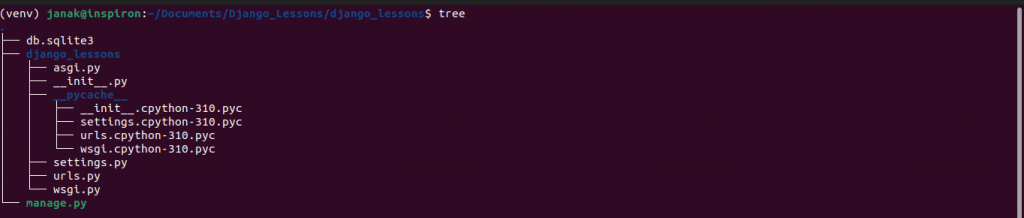
python3 manage.py runserver
Now, all warnings are gone.
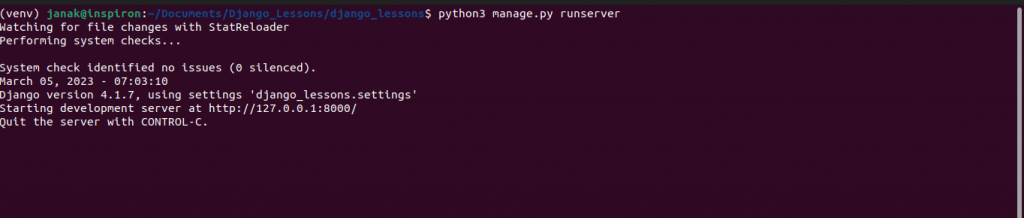
Visiting http://127.0.0.1:8000/
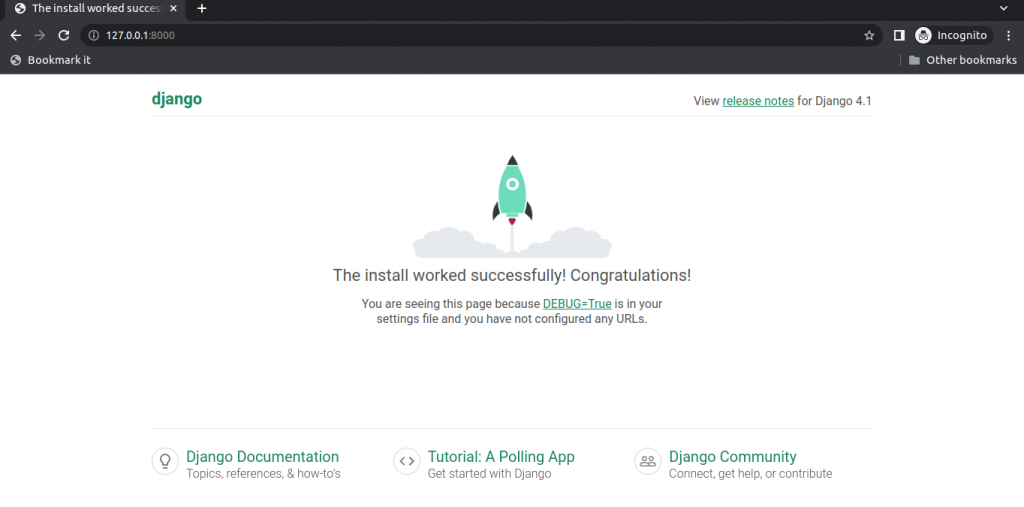
The Django MVT (Model-View-Template) pattern is as follows:
- Model : Manages data and core business logic
- View : Describes which data is sent to the user but not its presentation
- Template : Presents the data as HTML with optional CSS, JavaScript and Static Assets
- URL Configuration : Regular-expression components configured to a view
When you type in a URL, such as https://djangoproject.com, the first thing that happens within our Django project is a URL pattern (contained in urls.py) is foun that matches it. The URL pattern is linked to a single view (contained in views.py) which combines the data from the model (stored in models.py) and the styling from a template (any file ending in .html). The views then returns a HTTP response to the user.
The complete Django flow looks like this:
HTTP Request -> URL -> View -> Model and Templates -> HTTP Response
Create An App
Django uses the concept projects and apps to keep code clean and readable. A single top-level Django project can contain multiple apps. Each app controls an isolated piece of functionality. For example, an e-commerce site might have one app for user authentication, another app for payments and a third app to power item listing details. That’s three distinct apps that all live within one top-level project. How and when you split functionality into apps is somewhat subjective, but in general, each app should have a clear function.
To add a new app go to the command line and quit the running server with control+c. Then use the following command.
python3 manage.py startapp pages
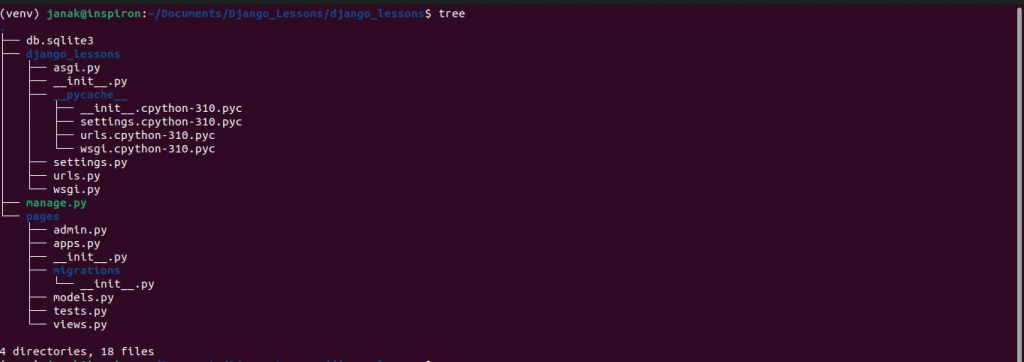
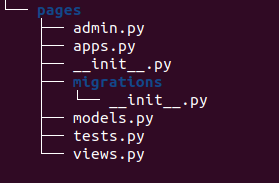
Let’s review what each new pages app file does:
- admin.py is a configuration file for the built-in Django Admin app
- apps.py is a configuration file for the app itself
- migrations/ keeps track of any changes to our models.py file so it stays in sync with our database
- models.py is where we define our database models which Django automatically translates into database tables
- tests.py is for app-specific tests
- views.py is where we handle the request/response logic for our web app
Notice that the model and view from the MVT pattern are present from the beginning. The url and template are missing which we will add shortly.
Even though our new app exists within the Django porject, Django does not know about it until we explicitly add it to the django_lessons/settings.py file.
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'pages.apps.PagesConfig',
]
PagesConfig is the name of function within pages/apps.py file at this point.
#inside pages/apps.py
from django.apps import AppConfig
class PagesConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'pages'
Hello Django
In Django, four separate files aligning with this MVT pattern are required to power one single dynamic (aka linked to a database) webpage.
- models.py
- views.py
- template.html (any HTML file will do)
- urls.py
However, to create a static webpage we can hardcode the data into a view so the model is not needed.
To create our first view we will update views.py file in our pages app as follows:
#pages/views.py
from django.http import HttpResponse
def homePageView(request):
return HttpResponse("Hello Django")
We’ve imported the built-in HttpResponse method so we can return a response object to the user. We’ve created a function called homePageView that accepts the request object and returns a response with the string “Hello Django”.
We need to configure our URLs. Create a new file called urls.py within the pages app. Then update it with the following code:
#pages/urls.py
from django.urls import path
from .views import homePageView
urlpatterns = [
path("",homePageView,name="home"),
]
On the top line we import path from Django to power our URL pattern and on the next line we import our views. By referring to the views.py file as .views we are telling Django to look within the current directory for views.py file and import the view homePageView from there.
Our URL pattern has three parts:
- a Python regular expression for the empty string ” “
- a reference to the view called homePageView
- an optional named URL pattern called “home”
In other words, if the user requests the homepage represented by the empty ” “, Django should use the view called homePageView.
The last step is to update our django_lessons/urls.py file. It’s commone to have multiple apps within a single Django project, like pages here, and they each need their own dedicated URL path.
#django_lessons/urls.py
from django.contrib import admin
from django.urls import path,include #new
urlpatterns = [
path("admin/",admin.site.urls),
path("",include("pages.urls")), #new
]
We have imported include on the second line next to path and then created a new URL pattern for our pages app. Now whenever a user visits the homepage, they will first be routed to the pages app and then to the homePageView view set in the pages/urls.py file.
Run the django again
python3 manage.py runserver
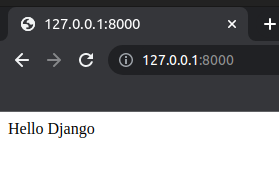
Putting code on Github
Create a new Repository
Clone
#git clone [repository URL]
git clone "git@github.com:gunjanak/Django_lessons.git"
Edit
pip3 freeze > requirements.txt
Push
git add .
git commit -m "Lesson one done"
git push