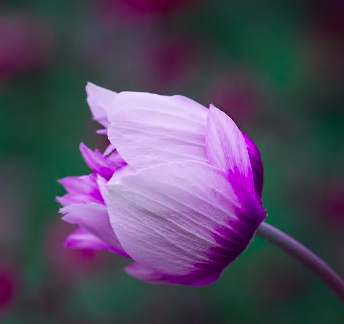
Flask with Jinja
Headline Example
In the following example, we display RSS feed of one of seven news agency as Headline. We use the ‘Jinja’ to form html template and display the dynamic data.
In the following we use the ‘feedparser’ library to format the ‘RSS feed’ and use it with python.
Following code contains the dictionary of seven news agency also with url of their RSS feed. We have used dynamic routing to pass the value of news agency and the data are fetched accordingly. Fetched data of selected news agency are passed dynamically to the ‘headline.html’ dynamically with the help of ‘Jinja’.
‘fox’ is selected as the default news agency in the following code.
import feedparser
from flask import Flask,render_template
app = Flask(__name__)
RSS_FEEDS = {'bbc':"http://feeds.bbci.co.uk/news/rss.xml",
'cnn':'http://rss.cnn.com/rss/edition.rss',
'fox':'http://feeds.foxnews.com/foxnews/latest',
'iol':'http://www.iol.co.za/cmlink/1.640',
'DW':"https://rss.dw.com/rdf/rss-en-all",
'dta':"https://www.dta.gov.au/news-blogs/feed/blog_post",
'France24':"https://www.france24.com/en/france/rss"}
@app.route("/")
@app.route("/<publication>")
def get_news(publication="fox"):
feed = feedparser.parse(RSS_FEEDS[publication])
first_article = feed['entries'][0]
title=first_article.get("title")
published = first_article.get("published")
summary = first_article.get("summary")
return render_template("headline.html",title=title,published=published,summary=summary)
if __name__ == '__main__':
app.run(port=5000,debug=True)
Code for ‘headline.html’
Double braces, {{ }}, indicate to Jinja that anything inside them should not be taken as literal HTML code. Because our placeholders, title, published, and summary, are the same as our Python variable names passed into the render_template call, just adding the surrounding braces means that the render_template call will substitute these for the real data, returning a pure HTML page.
<!DOCTYPE html>
<html>
<body>
<h1>Headlines</h1>
<b>{{ title }}</b>
<i>{{ published }}</i>
<p>{{ summary }}</p>
</body>
</html>
Output


Editing the above example to pass only one argument to html page.
All of the basic Python data structures, such as variables, objects, lists, and dictionaries, can be understood by Jinja and can be processed in a very similar way to what we are used to in Python.
For example, instead of passing each of the three components of our article separately to our template, we could have passed in the first_article object and dealt with the separation in Jinja.
import feedparser
from flask import Flask,render_template
app = Flask(__name__)
RSS_FEEDS = {'bbc':"http://feeds.bbci.co.uk/news/rss.xml",
'cnn':'http://rss.cnn.com/rss/edition.rss',
'fox':'http://feeds.foxnews.com/foxnews/latest',
'iol':'http://www.iol.co.za/cmlink/1.640',
'DW':"https://rss.dw.com/rdf/rss-en-all",
'dta':"https://www.dta.gov.au/news-blogs/feed/blog_post",
'France24':"https://www.france24.com/en/france/rss"}
@app.route("/")
@app.route("/<publication>")
def get_news(publication="fox"):
feed = feedparser.parse(RSS_FEEDS[publication])
first_article = feed['entries'][0]
title=first_article.get("title")
published = first_article.get("published")
summary = first_article.get("summary")
return render_template("headline.html",article = first_article)
if __name__ == '__main__':
app.run(port=5000,debug=True)
<!DOCTYPE html>
<html>
<body>
<h1>Headlines</h1>
<b>{{ article.title }}</b>
<i>{{ article.published}}</i>
<p>{{ article.summary }}</p>
</body>
</html>
Output
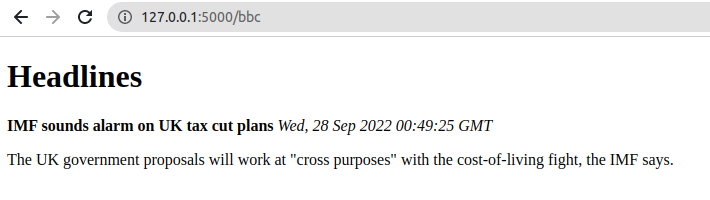
Adding looping logic to our template
We can make the whole list of articles available to Jinja
import feedparser
from flask import Flask,render_template
app = Flask(__name__)
RSS_FEEDS = {'bbc':"http://feeds.bbci.co.uk/news/rss.xml",
'cnn':'http://rss.cnn.com/rss/edition.rss',
'fox':'http://feeds.foxnews.com/foxnews/latest',
'iol':'http://www.iol.co.za/cmlink/1.640',
'DW':"https://rss.dw.com/rdf/rss-en-all",
'dta':"https://www.dta.gov.au/news-blogs/feed/blog_post",
'France24':"https://www.france24.com/en/france/rss"}
@app.route("/")
@app.route("/<publication>")
def get_news(publication="fox"):
feed = feedparser.parse(RSS_FEEDS[publication])
return render_template("headline.html",articles = feed['entries'])
if __name__ == '__main__':
app.run(port=5000,debug=True)
<!DOCTYPE html>
<html>
<body>
<h1>Headlines</h1>
{% for article in articles %}
<b>{{ article.title }}</b> </br>
<i>{{ article.published}}</i> </br>
<p>{{ article.summary }} </p> </br>
<hr/>
{% endfor %}
</body>
</html>
We can see that the Jinja for loop is similar to Python. It loops through the articles list that we’ve passed in from the Python code, and creates a new variable, article, for each iteration of the loop, each time referring to the next item in the list. The article variable can then be used like any other Jinja variable (using the double braces). Because whitespace in Jinja is irrelevant, unlike Python, we must define where
our loop ends with the {% endfor %} line. Finally, the “<hr/>”in HTML creates a horizontal line which acts as a separator between each article.
Output
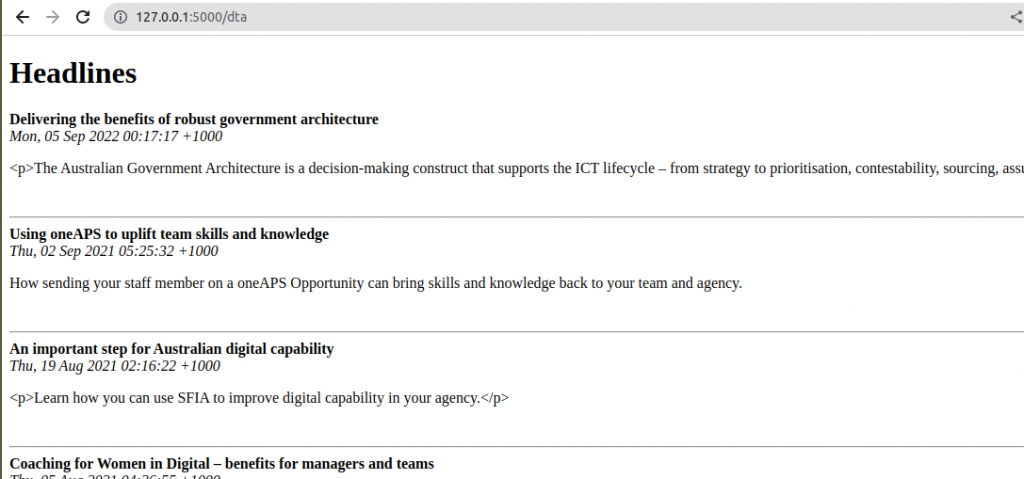
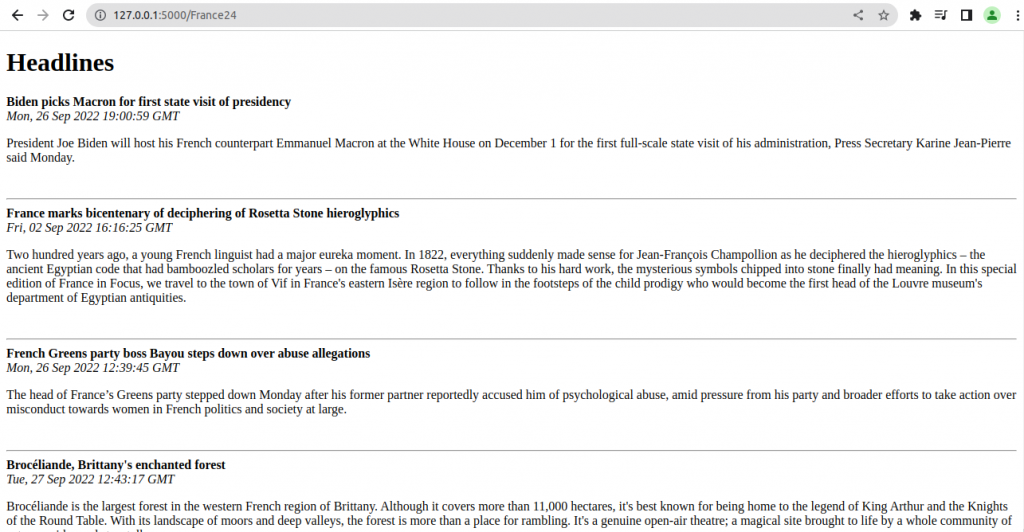
Adding Hyperlink to the template
Changing the html code to
<!DOCTYPE html>
<html>
<body>
<h1>Headlines</h1>
{% for article in articles %}
<b><a href="{{article.link}}">{{ article.title }}</a></b><br/>
<i>{{ article.published}}</i> </br>
<p>{{ article.summary }} </p> </br>
<hr/>
{% endfor %}
</body>
</html>
Output
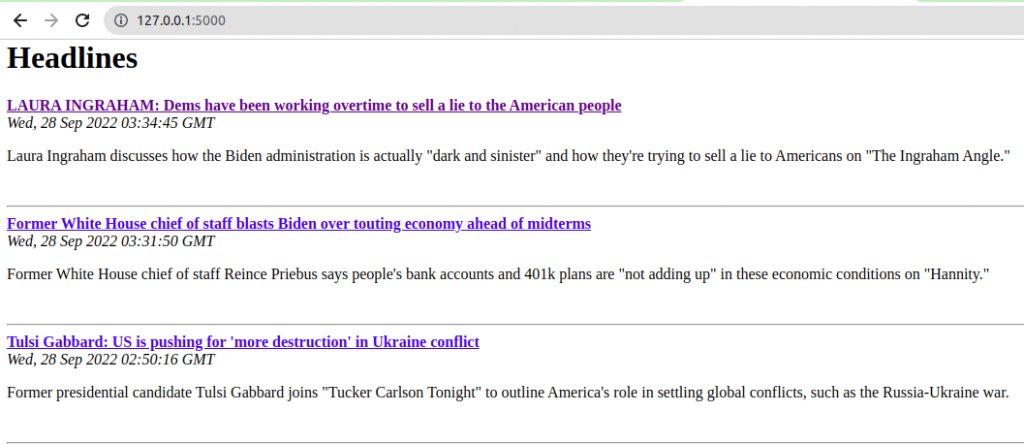
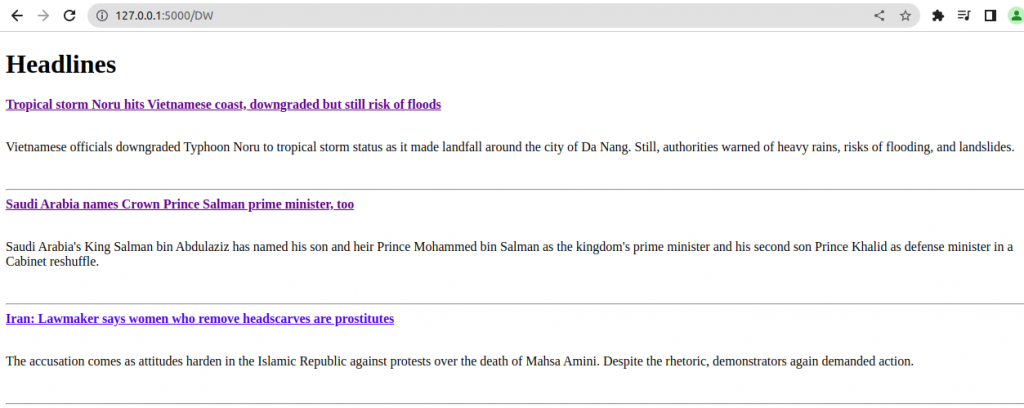