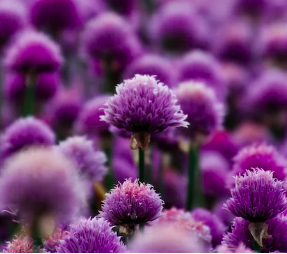
Flask and Cookies
We will ask the user his/her name, the city (for weather) and the news agency (for RSS feeds). Next time when the same user or someone uses the site using same browser from same data, we will show them customized information using previous data.
HTTP cookies (also called web cookies, Internet cookies, browser cookies, or simply cookies) are small blocks of data created by a web server while a user is browsing a website and placed on the user’s computer or other device by the user’s web browser. Cookies are placed on the device used to access a website, and more than one cookie may be placed on a user’s device during a session.
Basic Import For Cookies
import datetime
from flask import make_response
Flask’s make_response() function will help us to create a response object that we can set cookies on.
First, we will wrap a make_response() call around our render_template() call instead of returning the rendered template directly.
This means that our Jinja templates will be rendered, and all the placeholders will be replaced with the correct values, but instead of returning this response directly to our users, we will load it into a variable so that we can make some more additions to it. Once we have this response object, we will create a datetime object with a value of 365 days from today’s date. Then, we will do a series of set_cookie() calls on our response object, saving all the user’s selections (or refreshing the previous defaults)
and setting the expiry time to a year from the time the cookie was set using our datetime object.
Code for weather.py
import json
import json
from unittest.mock import DEFAULT
from urllib import response
import urllib3
import urllib
from urllib.parse import quote
import urllib.request as urll
import feedparser
from flask import Flask,render_template, make_response
from flask import request
import datetime
app = Flask(__name__)
RSS_FEEDS = {'bbc':"http://feeds.bbci.co.uk/news/rss.xml",
'cnn':'http://rss.cnn.com/rss/edition.rss',
'fox':'http://feeds.foxnews.com/foxnews/latest',
'iol':'http://www.iol.co.za/cmlink/1.640',
'dw':"https://rss.dw.com/rdf/rss-en-all",
'dta':"https://www.dta.gov.au/news-blogs/feed/blog_post",
'france24':"https://www.france24.com/en/france/rss"}
DEFAULTS = {'publication':'dta',
'city':'LONDON,UK',
'name':'Nusrat'}
def get_news(query):
if not query or query.lower() not in RSS_FEEDS:
publication = DEFAULTS["publication"]
else:
publication = query.lower()
feed = feedparser.parse(RSS_FEEDS[publication])
#print(feed)
return feed['entries']
def get_weather(query):
api_url = "http://api.openweathermap.org/data/2.5/weather?q={}&units=metric&appid=cb932829eacb6a0e9ee4f38bfbf112ed"
query = quote(query)
url = api_url.format(query)
data = urll.urlopen(url).read()
parsed = json.loads(data)
weather = None
if parsed.get("weather"):
weather = {"description":parsed["weather"][0]["description"],
"temperature":parsed["main"]["temp"],
"city":parsed["name"]}
return weather
@app.route("/",methods=['GET','POST'])
def home():
publication = request.form.get('publication')
#If publication is False, then value of "publication" from saved cookies is used.
#If there is not any value for "publication" in saved cookies then default value of "publication" is used
if not publication:
publication = request.cookies.get("publication")
if not publication:
publication = DEFAULTS['publication']
#Get articles from selected publication
articles = get_news(publication)
city = request.form.get('city')
#If "city" is False, then value of "city" from saved cookies is used.
#If there is not any value for "city" in saved cookies then default value of "city" is used
if not city:
city = request.cookies.get("city")
if not city:
city = DEFAULTS['city']
#Get weather of that city
weather = get_weather(city)
name = request.form.get('name')
if not name:
name = request.cookies.get('name')
if not name:
name = DEFAULTS['name']
print(name)
#print(request.cookies.get("name"))
response = make_response(render_template('weather.html',articles=articles,weather=weather,name=name))
#Setting Cookies
expires = datetime.datetime.now()+datetime.timedelta(days=365)
response.set_cookie("publication",publication,expires=expires)
response.set_cookie("city",city,expires=expires)
response.set_cookie("name",name,expires=expires)
return response
if __name__ == '__main__':
app.run(port=5000,debug=True)
Code for weather.html
<!DOCTYPE html>
<html>
<body>
<h2>Hello {{ name }}</h2>
<form action="/" method="POST">
<input type="text" name="name" placeholder="Your Name"/></br>
<label for="publication">Choose media house:</label></br>
<select name="publication" id="publication">
<option value="none" selected disabled hidden>Select a Media</option>
<option value="bbc">BBC</option>
<option value="cnn">CNN</option>
<option value="fox">Fox</option>
<option value="iol">IOL</option>
<option value="dw">DW</option>
<option value="dta">DTA</option>
<option value="france24">France 24</option>
</select>
</br>
<input type="text" name="city" placeholder="weather search"></br>
<input type="submit" value="Submit"/>
</form>
<p>City: <b> {{ weather.city }}</b></p>
<p>{{ weather.description }} | {{ weather.temperature }} ℃</p>
<h3>Headlines</h3>
{% for article in articles %}
<b><a href="{{article.link}}">{{ article.title }}</a></b><br/>
<i>{{ article.published}}</i> </br>
<p>{{ article.summary }} </p> </br>
<hr/>
{% endfor %}
</body>
</html>
Output
Default
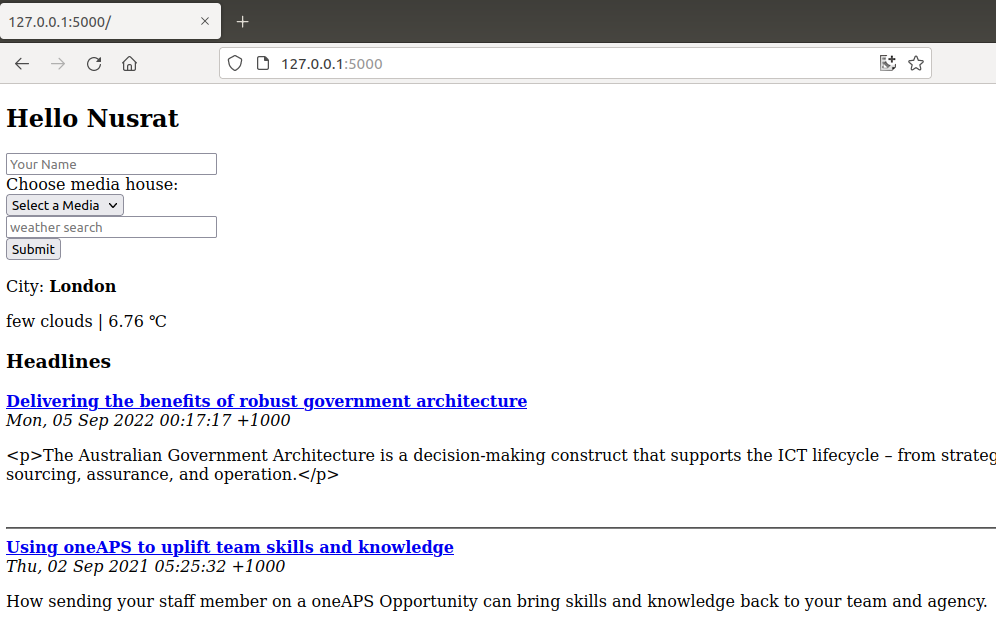
Entering some values
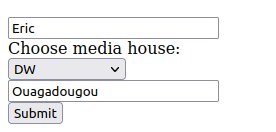
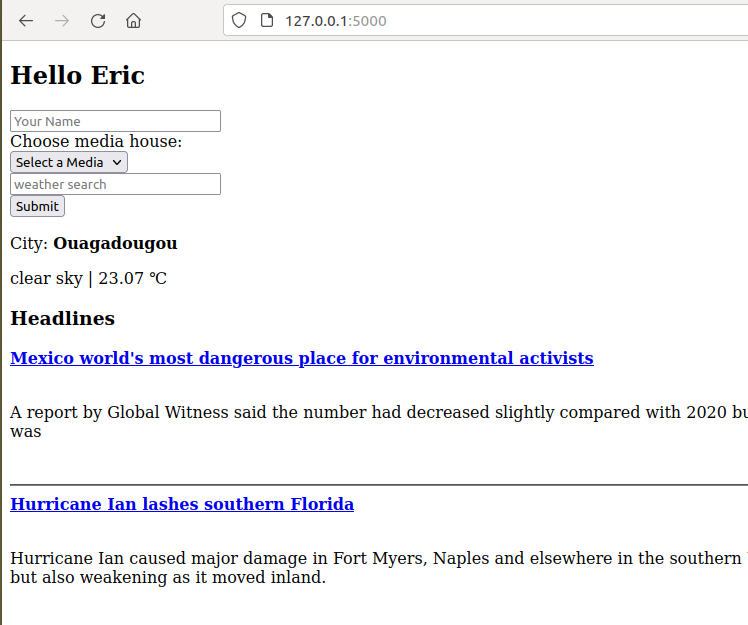
Revisiting The site
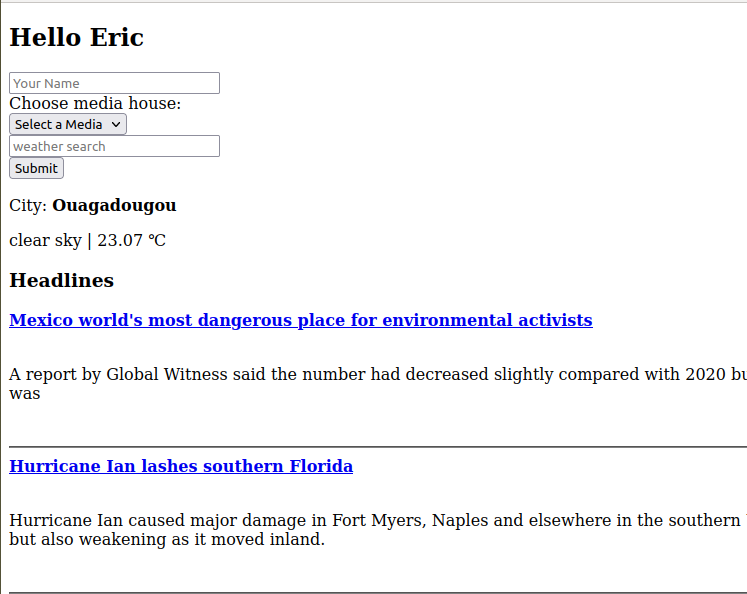
Entering Different Name and Media and leaving the city for weather search field empty
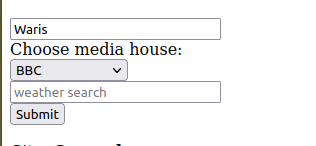
The shows the weather information of city that was entered previously
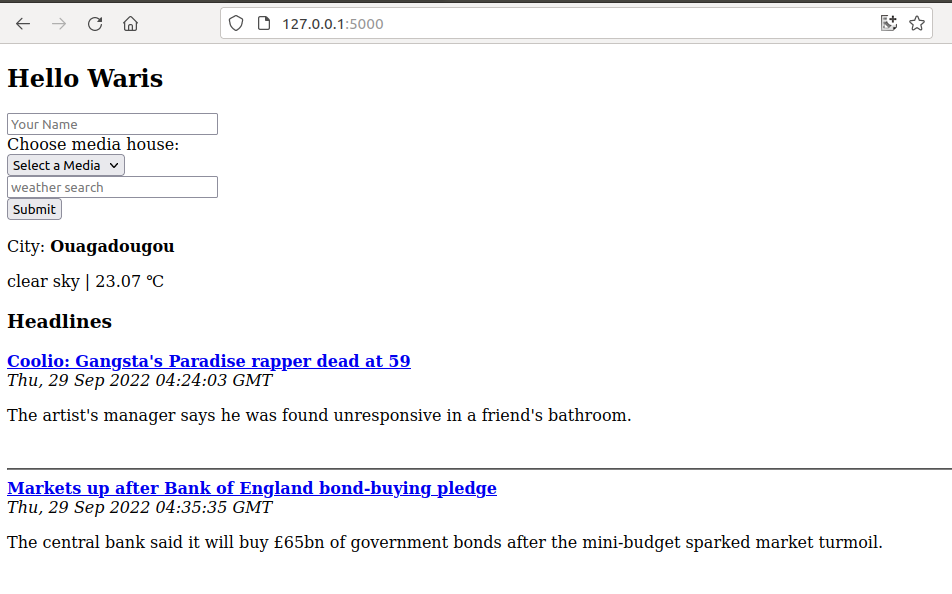