Django
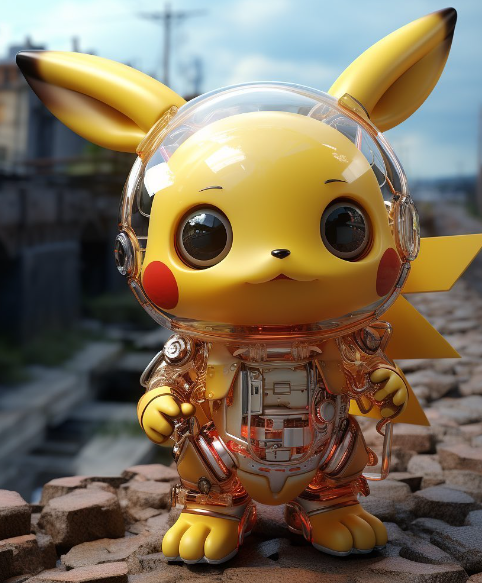
Django On MySql
In this article we will learn how to use MySql with Django. Operating system used in this process in Ubuntu 22.04.
For installation of MySql on Ubuntu 22.04 check this article.
Open terminal.
sudo mysql -u root -p
mysql>SHOW DATABASES;
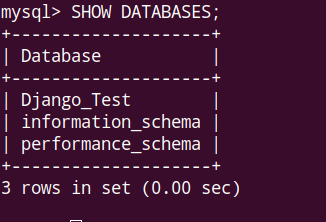
mysql> CREATE DATABASE Django_Blog;
mysql> SHOW DATABASES;
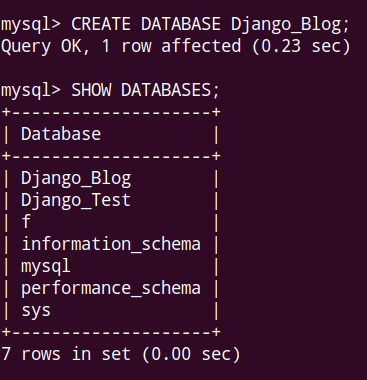
mysql> CREATE USER 'djangouser'@'localhost' IDENTIFIED WITH mysql_native_password BY 'Passwordofuser';
mysql> GRANT ALL IN Django_Blog.* TO 'djangouser'@'localhost';
mysql> FLUSH PRIVILEGES;
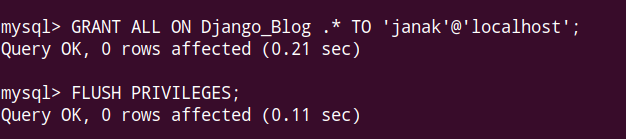
Exit Mysql.
sudo systemctl daemon-reload
sudo systemctl restart mysql
mkdir Django_MySql
cd Django_MySql
python3 -m venv venv
source venv/bin/activate
Install Django
pip install Django
Create a Django Project
django-admin startproject Django_MySql_Blog .
Basic installation
sudo apt install pkg-config
sudo apt install libmysqlclient-dev default-libmysqlclient-dev
pip install wheel
pip install mysqlclient
Create a Django application
python manage.py startapp blog
Edit Settings
cd Django_MySql_Blog
Open settings.py file and edit it
import os
....................................
# Application definition
INSTALLED_APPS = [
'blog',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
.......................................................
# DATABASES = {
# 'default': {
# 'ENGINE': 'django.db.backends.sqlite3',
# 'NAME': BASE_DIR / 'db.sqlite3',
# }
# }
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'Django_Blog',
'USER': 'djangouser',
'PASSWORD': 'Passwordofuser',
'HOST': 'localhost',
'PORT': '3306',
}
}
......................................................
STATIC_URL = 'static/'
STATIC_ROOT = os.path.join(BASE_DIR,'static')
Make Migrations and Migrate
python manage.py makemigrations
python manage.py migrate
Runserver
python manage.py runserver
Check the database being used
python manage.py dbshell
This will open console or shell of database being used (in this case mysql).
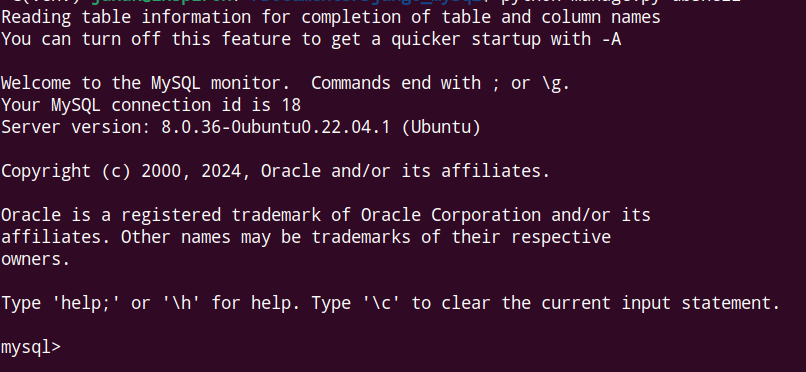
pontu
0
Tags :