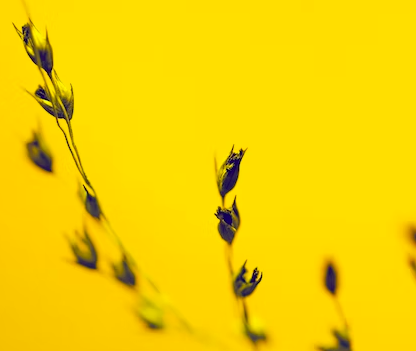
JavaScript Arrays
Table of Contents
- Creating Arrays
- Creating Empty Array
- Array with different datatypes as its element
- const Array
- Accessing elements of an Array
- Overwriting Elements of an Array
- Built-in length property
- Push
- splice()
- concat()
- pop()
- shift()
- delete
- Finding Elements
- indexof()
- Sorting and Reversing
- Multidimensional Array
Arrays
Creating Arrays
Rivers = [‘Mechi’,’Koshi’,’Sarda’’]
Cars = new Array(“Sedan”,”Maruti”,”Audi”)
console.log(Rivers)
console.log(Cars)
Output
[ ‘Mechi’, ‘Koshi’, ‘Sarda’ ]
[ ‘Sedan’, ‘Maruti’, ‘Audi’ ]
Creating Empty Array
Empty_array = new Array(10);
console.log(Empty_array)
Output
[ <10 empty items> ]
Array with different datatypes as its element
let arr = ['Mechi',123,true]
console.log(typeof arr[0])
console.log(typeof arr[1])
console.log(typeof arr[2])
Output
string
number
boolean
const Array
We can define an array using const. We can change the values of a constant array, bu we cannot change the array itself.
const car = ['Skoda']
car[0] = 'Audi'
console.log(car)
console.log(car[0])
car = ['Toyota']
Output:
[ ‘Audi’ ]
Audi
HelloWorld.js:6
car = [‘Toyota’]
^
TypeError: Assignment to constant variable.
Accessing Elements of an Array
Rivers = ['Mechi','Koshi','Kaveri','Narayani','Sarda','Teshio']
console.log(Rivers[0])
console.log(Rivers[1])
console.log(Rivers[-1])
console.log(Rivers[-2])
console.log(Rivers[4])
Output
Mechi
Koshi
undefined
undefined
Sarda
Overwriting Elements of an Array
Rivers = ['Mechi','Koshi','Kaveri','Narayani','Sarda','Teshio']
console.log(Rivers[0])
console.log(Rivers)
Rivers[0] = 'Abukuma'
Rivers[6] = 'Mogami'
console.log(Rivers)
Output
Mechi
[ ‘Mechi’, ‘Koshi’, ‘Kaveri’, ‘Narayani’, ‘Sarda’, ‘Teshio’ ]
[
‘Abukuma’, ‘Koshi’,
‘Kaveri’, ‘Narayani’,
‘Sarda’, ‘Teshio’,
‘Mogami’
]
Built-in length property
Rivers = ['Mechi','Koshi','Kaveri','Narayani','Sarda','Teshio'];
Movies = ['Titanic','Avatar','Inception','Julia'];
bathrobe = [];
console.log("length of Movies array: ",Rivers.length)
console.log("length of Movies array: ",Movies.length);
console.log("Length of bathrobe array: ",bathrobe.lenght)
Output
length of Movies array: 6
length of Movies array: 4
Length of bathrobe array: undefined
More example
Movies = ['Titanic','Avatar','Inception','Julia'];
console.log(Movies)
console.log("length of Movies array: ",Movies.length);
Movies[9] = 'Chopsticks'
console.log("length of Movies array: ",Movies.length);
console.log(Movies)
Output
[ ‘Titanic’, ‘Avatar’, ‘Inception’, ‘Julia’ ]
length of Movies array: 4
length of Movies array: 10
[”Titanic’,
‘Avatar’,
‘Inception’,
‘Julia’,
<5 empty items>,
‘Chopsticks’
]
Array Methods
Adding and replacing elements
We can add elements with the push() method.
writers = ['Murakami','Agatha','Sidney','Xin']
console.log(writers)
writers.push('Brown')
console.log(writers)
Output
[ ‘Murakami’, ‘Agatha’, ‘Sidney’, ‘Xin’ ]
[ ‘Murakami’, ‘Agatha’, ‘Sidney’, ‘Xin’, ‘Brown’ ]
splice() method
writers = ['Murakami','Agatha','Sidney','Xin']
console.log(writers)
writers.splice(2,0,'Dickens','Kafka')
console.log(writers)
Output
[ ‘Murakami’, ‘Agatha’, ‘Sidney’, ‘Xin’ ]
[ ‘Murakami’, ‘Agatha’, ‘Dickens’, ‘Kafka’, ‘Sidney’, ‘Xin’ ]
The splice() method takes multiple parameters. The first parameter, 2 in our case, is the index of the array on which we want to start inserting. The second parameter, 0 in our case, is the number of elements we want to delete starting at our previously defined starting index. The parameters after these first two, ‘Dickens’ and ‘Kafka’ in our case, are whatever should be inserted starting at the start index.
Another Example
writers = ['Murakami','Agatha','Sidney','Xin']
console.log(writers)
writers.splice(2,1,'Tolstoy','Wilde')
console.log(writers)
Output
[ ‘Murakami’, ‘Agatha’, ‘Sidney’, ‘Xin’ ]
[ ‘Murakami’, ‘Agatha’, ‘Tolstoy’, ‘Wilde’, ‘Xin’ ]
In second case one item gets deleted at index 2. If you were to increase the second parameter to a number higher than our array, it would not affect the result as JavaScript would simply stop as soon as it runs out of values to delete. Try the following code:
writers = ['Murakami','Agatha','Sidney','Xin']
console.log(writers)
writers.splice(1,13,'Tolstoy','Wilde')
console.log(writers)
Output
[ ‘Murakami’, ‘Agatha’, ‘Sidney’, ‘Xin’ ]
[ ‘Murakami’, ‘Tolstoy’, ‘Wilde’ ]
Deleting elements with splice()
russian_writers = ['Leo','Vladimir','Viktor','Mikhali','Leskov','Chekhov','Gorkey']
console.log(russian_writers)
russian_writers.splice(2,4)
console.log(russian_writers)
Output:
[”Leo’, ‘Vladimir’,’Viktor’, ‘Mikhali’,’Leskov’, ‘Chekhov’, ‘Gorkey’]
[ ‘Leo’, ‘Vladimir’, ‘Gorkey’ ]
We specify the index from where we want to start deleting and then the number of elements we want to delete.
concat()
We can also add one array to another with the concat method. This way, we can create a new array that consists of a concatenation of both arrays. The elements of the first array will be first, and the elements of the argument of concat() will be concatenated to the end.
russian_writers = ['Leo','Vladimir']
console.log(russian_writers)
french_writers = ['Hugo','Sand','Duras']
console.log(french_writers)
writers = russian_writers.concat(french_writers)
console.log(writers)
Output
[ ‘Leo’, ‘Vladimir’ ]
[ ‘Hugo’, ‘Sand’, ‘Duras’ ]
[ ‘Leo’, ‘Vladimir’, ‘Hugo’, ‘Sand’, ‘Duras’ ]
We can use concat() method to add values. We can add a single value or multiple values separated by comma.
russian_writers = ['Leo','Vladimir']
console.log(russian_writers)
more_russian_writers = russian_writers.concat('Viktor')
console.log(more_russian_writers)
more_russian_writers = more_russian_writers.concat('Mikhali','Leskov')
console.log(more_russian_writers)
Output
[ ‘Leo’, ‘Vladimir’ ]
[ ‘Leo’, ‘Vladimir’, ‘Viktor’ ]
[ ‘Leo’, ‘Vladimir’, ‘Viktor’, ‘Mikhali’, ‘Leskov’ ]
Delete elements with pop()
russian_writers = ['Leo','Vladimir','Viktor','Mikhali','Leskov']
console.log(russian_writers)
russian_writers.pop()
console.log(russian_writers)
Output
[ ‘Leo’, ‘Vladimir’, ‘Viktor’, ‘Mikhali’, ‘Leskov’ ]
[ ‘Leo’, ‘Vladimir’, ‘Viktor’, ‘Mikhali’ ]
shift()
Deleting the first element can be done with shift(). This causes all other indices to be reduced by one.
russian_writers = ['Leo','Vladimir','Viktor','Mikhali','Leskov']
console.log(russian_writers[1])
russian_writers.shift()
console.log(russian_writers[1])
Output
Vladimir
Viktor
delete
russian_writers = ['Leo','Vladimir','Viktor','Mikhali','Leskov','Chekhov','Gorkey']
delete russian_writers[0]
console.log(russian_writers)
delete russian_writers[3]
console.log(russian_writers)
delete russian_writers
console.log(russian_writers)
Output
[<1 empty item>,’Vladimir’,’Viktor’,’Mikhali’, ‘Leskov’, ‘Chekhov’, ‘Gorkey’]
[ <1 empty item>, ‘Vladimir’, ‘Viktor’, <1 empty item>, ‘Leskov’, ‘Chekhov’, ‘Gorkey’]
console.log(russian_writers)
^
ReferenceError: russian_writers is not defined
Finding Elements
If we want to check whether a value is present in an array, we can use the find() method.
find() takes a function.
prime_num = [2,3,5,7,11,13,17]
let find1 = prime_num.find(function(w) {
return w == 7
});
console.log(find1)
let find2 = prime_num.find(w => w === 13);
console.log(find2)
Output
7
13
Finding index of elements with indexof()
It returns the position of the element.
prime_num = [2,3,5,7,11,13,17]
let findIndex = prime_num.indexOf(13)
console.log(findIndex)
Output
5
Another example of indexof()
prime_num = [2,3,5,7,11,13,17]
let findIndex = prime_num.indexOf(33)
console.log(findIndex)
Output
-1
In this case, since the array does not contain ‘33’. The indexOf function returns -1.
If we want to find the next occurrence of the specified number, we can add a second argument of indexOf(), specifying from which position it should start searching:
menu = ['pizza','pasta','pizza','fries','salad','dumpling']
let findIndex = menu.indexOf('pizza',3)
console.log(findIndex)
findIndex = menu.indexOf('pizza',1)
console.log(findIndex)
Output
-1
2
The last occurrence of an element can be found with the lastIndexOf() method.
menu = ['pizza','pasta','pizza','fries','salad','dumpling']
let lastIndex = menu.lastIndexOf('pizza')
console.log(lastIndex)
Output
2
Sorting and Reversing
menu = ['pizza','pasta','pizza','fries','salad','dumpling']
menu.sort()
console.log(menu)
Output
[ ‘dumpling’, ‘fries’, ‘pasta’, ‘pizza’, ‘pizza’, ‘salad’ ]
The elements of the array can be reversed by calling the built-in method, reverse(), on an array. It puts the last element first, and the first element last. It does not matter whether the array is sorted or not.
menu = ['pizza','pasta','pizza','fries','salad','dumpling']
menu.reverse()
console.log(menu)
Output
[ ‘dumpling’, ‘salad’, ‘fries’, ‘pizza’, ‘pasta’, ‘pizza’ ]
Multidimensional Array
russian = ['Tolstoy','Gogol','Chekhov']
japanese = ['Murakami','Mishima','Kawakami']
nigerian = ['Orki','Habila','Tutuola']
writers= [russian,japanese,nigerian]
console.log(writers)
Output:
[
[ ‘Tolstoy’, ‘Gogol’, ‘Chekhov’ ],
[ ‘Murakami’, ‘Mishima’, ‘Kawakami’ ],
[ ‘Orki’, ‘Habila’, ‘Tutuola’ ]
]
Accessing elements of multidimensional array
russian = ['Tolstoy','Gogol','Chekhov']
japanese = ['Murakami','Mishima','Kawakami']
nigerian = ['Orki','Habila','Tutuola']
writers= [russian,japanese,nigerian]
console.log(writers[0])
console.log(writers[1][2])
Output:
[ ‘Tolstoy’, ‘Gogol’, ‘Chekhov’ ]
Kawakami
3D Array
array1 = [[1,2],[3,4]]
array2 = [[5,6],[7,8]]
array3 = [[9,10],[11,12]]
array = [array1,array2,array3]
console.log(array)
Output:
[
[ [ 1, 2 ], [ 3, 4 ] ],
[ [ 5, 6 ], [ 7, 8 ] ],
[ [ 9, 10 ], [ 11, 12 ] ]
]
array1 = [[1,2],[3,4]]
array2 = [[5,6],[7,8]]
array3 = [[9,10],[11,12]]
array = [array1,array2,array3]
console.log(array[0])
Output
[ [ 1, 2 ], [ 3, 4 ] ]
array1 = [[1,2],[3,4]]
array2 = [[5,6],[7,8]]
array3 = [[9,10],[11,12]]
array = [array1,array2,array3]
console.log(array[2][1])
Output:
[ 11, 12 ]
array1 = [[1,2],[3,4]]
array2 = [[5,6],[7,8]]
array3 = [[9,10],[11,12]]
array = [array1,array2,array3]
console.log(array[2][1][1])
Output:
12
array1 = [[1,2],[3,4]]
array2 = [[5,6],[7,8]]
array3 = [[9,10],[11,12]]
array = [array1,array2,array3]
console.log(array[2][2])
Output:
undefined