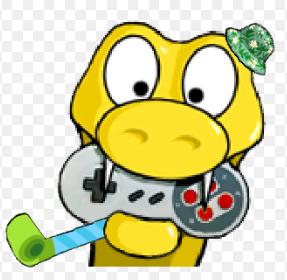
Introduction To Python Games
This is an introduction to Python Games with Pygame.
Table of Contents
Hello World with Pygame
import pygame,sys
from pygame.locals import *
pygame.init()
DISPLAYSURF = pygame.display.set_mode((400,300))
pygame.display.set_caption("Hello World")
#main game loop
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
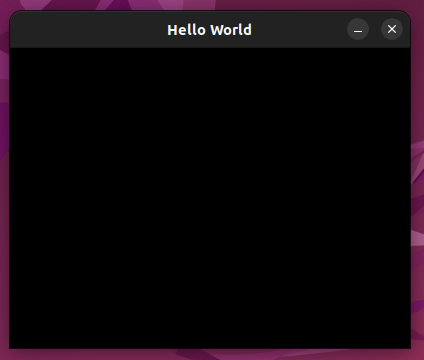
import pygame,sys
At first we imported the pygame and sys modules so that our program can use the functions in them. All of the Pygame functions dealing with graphics, sound and other features that Pygame provides are in the pygame module.
from pygame.locals import *
pygame.locals contains several constant variables such as MOUSEMOTION, QUIT, KEYUP etc.
pygame.init()
We need to call this function after importing the pygame module and before calling any other Pygame function.
DISPLAYSURF = pygame.display.set_mode((400,300))
pygame.display.set_mode((400,300)) returns the pygame.Surface object for the window. The tuple (400,300) tells the set_mode() function how wide and how high to make the window in pixels.
pygame.display.set_caption("Hello World")
Setting the caption to “Hello World”
#main game loop
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
This is the main loop of the game.
The for loop will iterate over the list of Event objects that was returned by pygame.event.get(). On each iteration through the for loop, a variable named event will be assigned the value of the next event object in this list. The list of Event objects returned from pygame.event.get() will be in the order that the events happened. If the user clicked the mouse and then pressed a keyboard key, the Event object for the mouse click would be the first item in the list and the Event object for the keyboard press would be second. If no events have happened, the pygame.event.get() will return a blank list.
If the event object is a quit event, then the pygame.quit() ans sys.exit() functions are called. The pygame.quit() function is sort of the opposite of the pygame.init() function: it runs the code that deactivates the Pygame library.
Primitive Drawing in Pygame
import pygame,sys
from pygame.locals import *
pygame.init()
#set up the window
DISPLAYSURF = pygame.display.set_mode((500,400),0,32)
pygame.display.set_caption('Drawing')
#set up the colors
BLACK = (0,0,0)
WHITE = (255,255,255)
RED = (255,0,0)
GREEN = (0,255,0)
BLUE = (0,0,255)
#draw on the surface object
DISPLAYSURF.fill(WHITE)
#Run the game loop
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
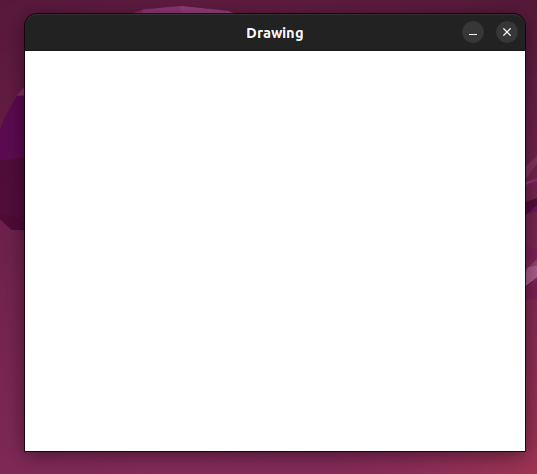
#add following lines of code in to draw a polygon
#Draw polygon
pygame.draw.polygon(DISPLAYSURF,GREEN,((146,0),(291,106),(236,277),(56,277),(0,106)))
pygame.draw.polygon(surface,color,pointlist,width)
The surface and color parameters tell the function on what surface to draw the polygon and what color to make it.
The pointlist parameter is a tuple or list of points (that is tuple or list of two integer tuples for XY coordinates). The polygon is drawn by drawing lines between each point and the point that comes after it in the tuple. Then a line is drawn from the last point to the first point. We can also point a list of points instead of a tuple of points.
The width parameter is optional. If you leave it out, the polygon that is drawn will be filled in, just like our green polygon on the screen. If you do pass an integer value for the width parameter, only the outline of the polygon will be drawn.
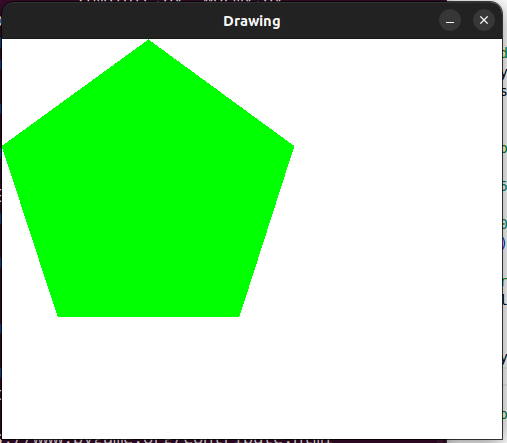
#Draw polygon with list of points and width == 5
pygame.draw.polygon(DISPLAYSURF,GREEN,[(146,0),(291,106),(236,277),(56,277),(0,106)],5)
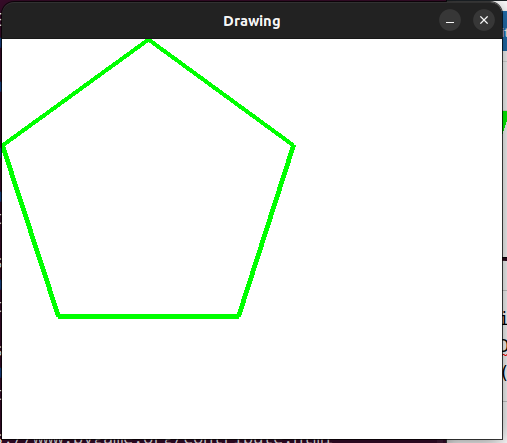
#Drawing lines
pygame.draw.line(DISPLAYSURF,BLUE,(60,60),(120,60),4)
This code will draw a blue line form (60,60) to (120,60) and have width of 4.
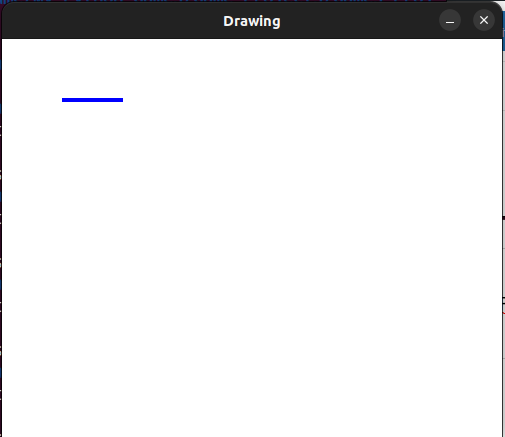
#Drawing a circle
pygame.draw.circle(DISPLAYSURF,RED,(30,50),20,0)
#Drawing another circle
pygame.draw.circle(DISPLAYSURF,ORANGE,(100,200),50,10)
pygame.draw.circle(surface,color,center_point,radius,width)
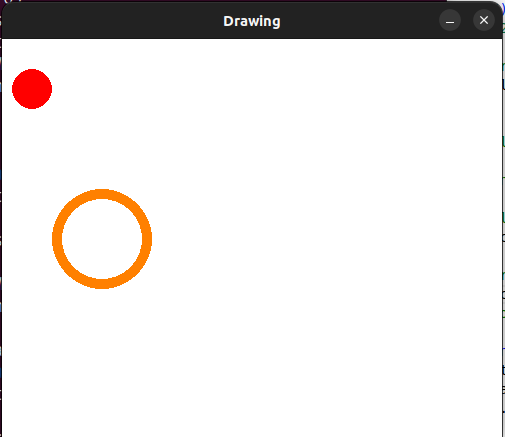
#Drawing ellipse
pygame.draw.ellipse(DISPLAYSURF,GREEN,(20,20,200,200),1)
pygame.draw.ellipse(DISPLAYSURF,GREEN,(220,10,250,30))
pygame.draw.ellipse(surface,color,bounding_rectangle,width).
In order to tell the function how large and where to draw the ellipse, we need to specify the bounding rectangle of the ellipse.
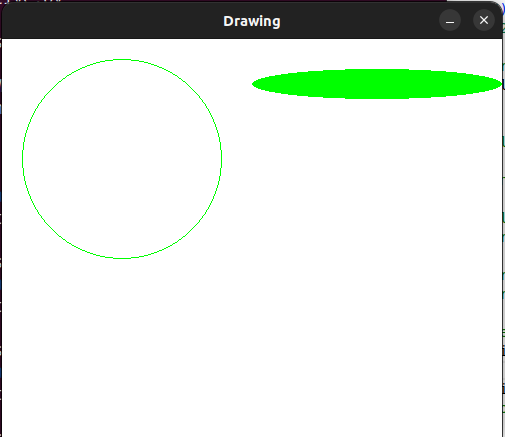
#Drawing rectangle
pygame.draw.rect(DISPLAYSURF,RED,(200,150,100,50))
The rectangle_tuple is either a tuple of four integers (for the XY coordinates of the top left corner, and the width and height) or a pygame.Rect.
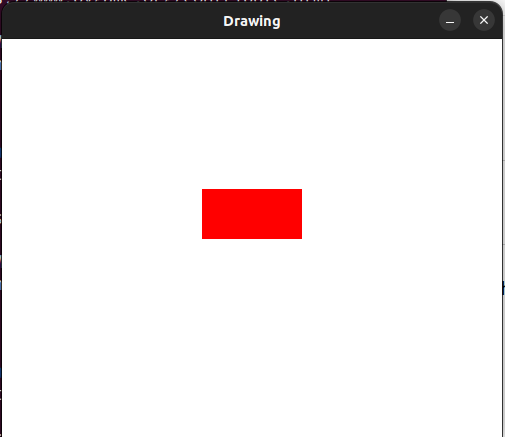
Basic Animation
A circle bouncing off the sides of the screen.
import pygame,sys
from pygame.locals import *
pygame.init()
#set up the window
DISPLAYSURF = pygame.display.set_mode((500,400),0,32)
pygame.display.set_caption('Animating a Circle')
#set up the circle
radius = 50
x = 50
y = 200
speed = 5
#Set up the colors
WHITE = (255,255,255)
RED = (255,0,0)
#The game loop
while True:
#handle the event
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
#Move the circle
x += speed
#Bounce the circle off the sides of the screen
if x+radius > 640 or x-radius < 0:
speed = - speed
#Draw the circle
DISPLAYSURF.fill(WHITE)
pygame.draw.circle(DISPLAYSURF,RED,(x,y),radius)
pygame.display.update()
This code will start with the circle of radius 50 at the position (50,20). The value of x is changed to to 50+speed in each iteration and new circle is drawn. This is continued until the circle finds the end of screen after that it moves towards another end.
Animating Background
The following code changes the background color in every 1/10th second.
import pygame,sys,random
from pygame.locals import *
pygame.init()
#set up the window
DISPLAYSURF = pygame.display.set_mode((500,400),0,32)
pygame.display.set_caption('Channging colors randomly')
#set up the circle
RED = 0
GREEN = 0
BLUE = 0
#The game loop
while True:
#handle the event
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
RED = random.randint(0, 255)
GREEN = random.randint(0, 255)
BLUE = random.randint(0, 255)
DISPLAYSURF.fill((RED,GREEN,BLUE))
pygame.time.wait(100)
pygame.display.update()
Keyboard Control
keys = pygame.key.get_pressed() detects the key and following series of if statements changes the center of circle accordingly.
import pygame
import sys
# Initialize Pygame
pygame.init()
# Set up the window
DISPLAYSURF = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Moving a Circle with Keyboard Arrows")
#colors
WHITE = (255,255,255)
RED = (255,0,0)
# Set up the circle
radius = 20
x = 320
y = 240
speed = 2
# Start the game loop
while True:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the circle according to keyboard arrows
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
x -= speed
if keys[pygame.K_RIGHT]:
x += speed
if keys[pygame.K_UP]:
y -= speed
if keys[pygame.K_DOWN]:
y += speed
# Draw the circle
DISPLAYSURF.fill(WHITE)
pygame.draw.circle(DISPLAYSURF,RED, (int(x), int(y)), radius)
pygame.display.update()
Mouse control in Pygame
import pygame
import sys,math
# Initialize Pygame
pygame.init()
# Set up the window
DISPLAYSURF = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Moving a Circle with Mouse Cursor")
# Set up the circle
radius = 50
x = 320
y = 240
speed = 5
#Set up colors
#colors
WHITE = (255,255,255)
RED = (255,0,0)
# Start the game loop
while True:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the circle according to the mouse cursor
mouse_pos = pygame.mouse.get_pos()
target_x, target_y = mouse_pos
dx = target_x - x
dy = target_y - y
distance = math.sqrt(dx**2 + dy**2)
if distance != 0:
x += dx / distance * speed
y += dy / distance * speed
# Draw the circle
DISPLAYSURF.fill(WHITE)
pygame.draw.circle(DISPLAYSURF,RED, (int(x), int(y)), radius)
pygame.time.wait(100)
pygame.display.flip()
mouse_pos = pygame.mouse.get_pos() to get the position of mouse cursor. The we calculate the distance between the mouse cursor’s position and current center of circle. Then we change the values of x and y accordingly.