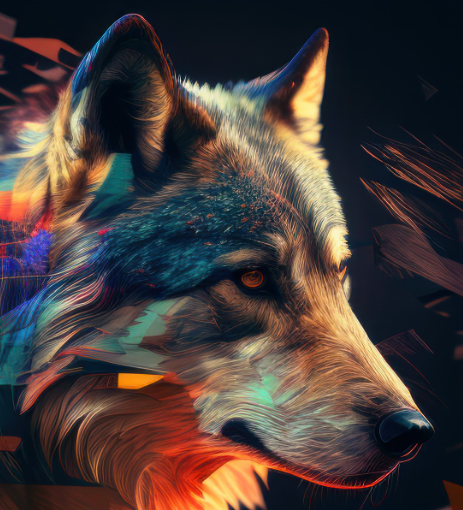
Blog App in Django Part III
In this final part of our Blog App tutorial we will add user log in, log out and sign up options.
Table of Contents
Log In
Django provides us with a default view for a log in page via
We need to update the django_blog_project/urls.py file so that we could place our login and log our pages at the /accounts/ URL. This is a one-line addition on the next-to-last line.
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
#new
path("accounts/",include("django.contrib.auth.urls")),
path("",include("blog.urls")),
]
Now, create a registration directory inside templates directory and within that registration directory create file login.html.
Fill templates/registration/login.html with following code
{% extends "base.html" %}
{% block content %}
<h2>Log In</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Log In</button>
</form>
{% endblock content %}
We are using HTML <form></form> tags and specifying the POST method since we are sending data to the server. We add {% csrf_token %} for security concerns, namely to prevent a CSRF Attack. The form’s contents are outputted between paragraph tags due to {{ form.as_p }} and the we add a “submit” button.
The final step is we need to specify where to redirect the user upon a successful log in. We can set this with the LOGIN_REDIRECT_URL setting. At the bottom of the django_blog_project/settings.py file add the following code.
#django_blog_project/settings.py
LOGIN_REDIRECT_URL = "home"
Now the user will be redirected to the “home” template which is our homepage.
Visit http://127.0.0.1:8000/accounts/login/ to see the login form.
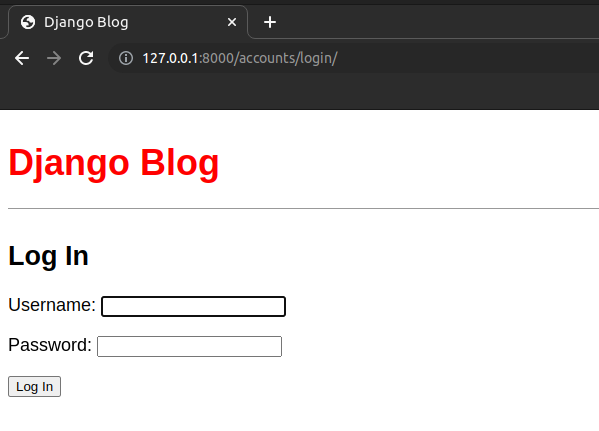
For now fill it with your superuser name and password.
Then you will be redirected to login field.
Update Homepage
We need to update our base.html template so we could display a message to users whether they are logged in or not. We can use the is_authenticated attribute for this.
Update templates/base.html as follow
...........................................
</header>
{% if user.is_authenticated %}
<p>Hi {{ user.username }}!</p>
{% else %}
<p>You are not logged in </p>
<a href="{% url 'login' %}">Log In</a>
{% endif %}
<div>
{% block content %}
{% endblock content %}
</div>
If the user is logged in the we display “Hi <user>”
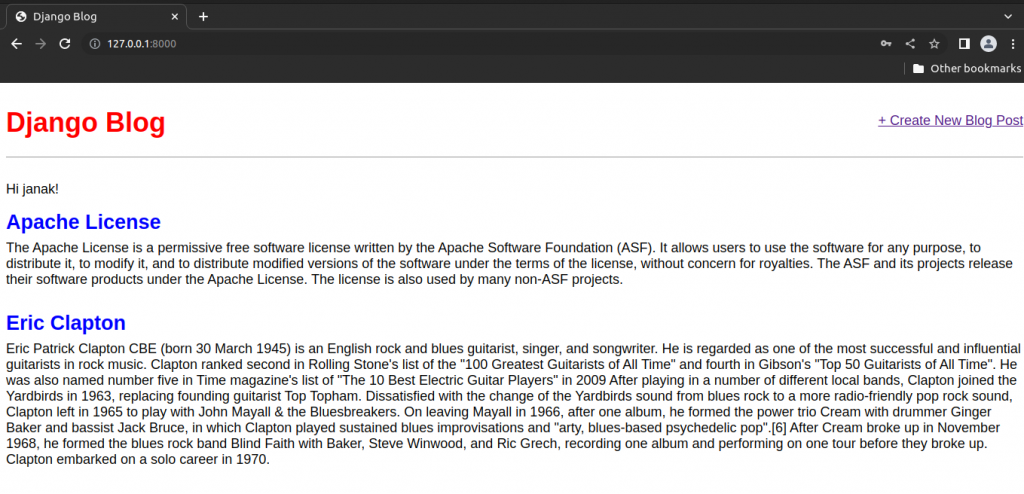
If the user is not logged in then we provide them link to log-in.
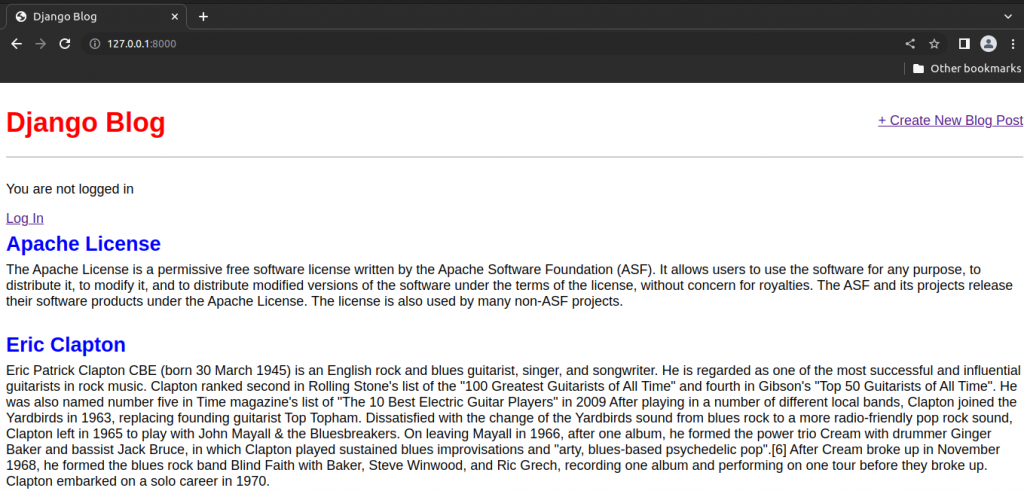
Log Out Link
We need to provide a logout link so that our user can logout.
In our base.html file add a one-line {% url ‘logout’ %} link for logging out just below our user greeting.
That’s all we need to do as the necessary view is provided to us by the Django auth app. We do need to specify where to redirect a user log out though.
Update django_blog_project/settings.py to provide a redirect link which is called, LOGOUT_REDIRECT_URL.
#django_blog_project/settings.py
.....................................
LOGIN_REDIRECT_URL = "home"
LOGOUT_REDIRECT_URL = "home" #new
Run the server and login.
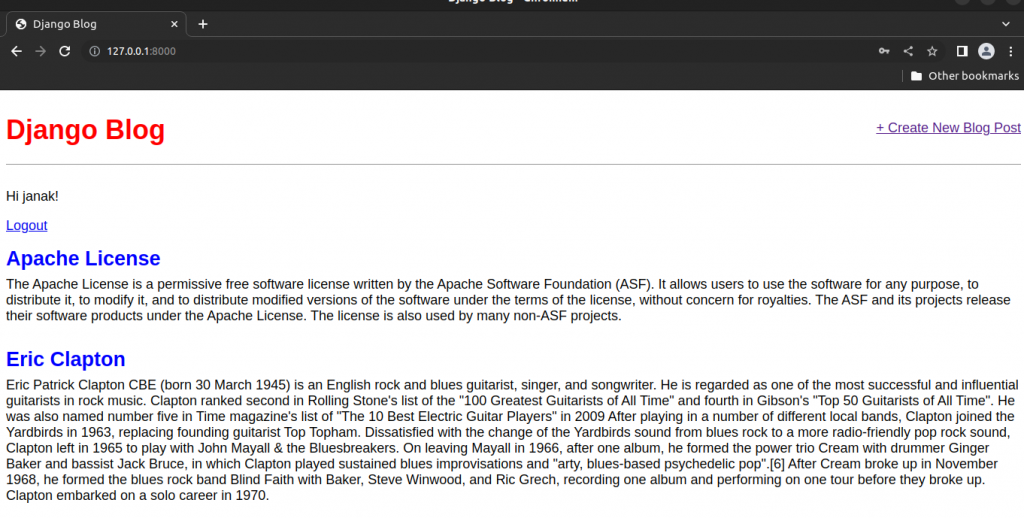
Now we have Logout option. After clicking the Logout we are redirected to home page.
Sign Up
We will use UserCreationForm for the Sign Up. Create a dedicated new app, accounts, for our sign up page.
#Terminal
python3 manage.py startapp accounts
Add the new app to the INSTALLED_APPS setting in our django_blog_app/settings.py file.
#django_blog_app/settings.py
...................
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
"blog.apps.BlogConfig",
"accounts.apps.AccountsConfig",#new
]
Now add a new URL path in django_blog_app/urls.py pointing to this new app directly below where we include the built-in auth app.
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path("accounts/",include("django.contrib.auth.urls")),
path("accounts/",include("accounts.urls")),#new
path("",include("blog.urls")),
]
The order of our urls matters here because Django reads this file top-to-bottom. Therefore when we request the /accounts/signup url, Django will first look in auth, not find it and then proceed to the accounts app.
Now, create a file called accounts/urls.py and add the following code.
#accounts/urls.py
from django.urls import path
from .views import SignUpView
urlpatterns = [
path('signup/',SignUpView.as_view(),name="signup"),
]
We will now create SignUpView class in our accounts/views.py
from django.contrib.auth.forms import UserCreationForm
from django.urls import reverse_lazy
from django.views.generic import CreateView
# Create your views here.
class SignUpView(CreateView):
form_class = UserCreationForm
success_url = reverse_lazy("login")
template_name = "registration/signup.html"
We created SignUpView class which is a subclass of CreateView. We specify the use of the built-in UserCreationForm and the not-yet-created template at signup.html. And we use reverse_lazy to redirect the user to the log in page upon successful registration.
Now create the file signup.html file within templates/registration directory and fill it will following code.
{% extends "base.html" %}
{% block content %}
<h2>Sign Up</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Sign Up</button>
</form>
{% endblock content %}
Visit http://127.0.0.1:8000/accounts/signup/ after running the server. You will find the following signup form.
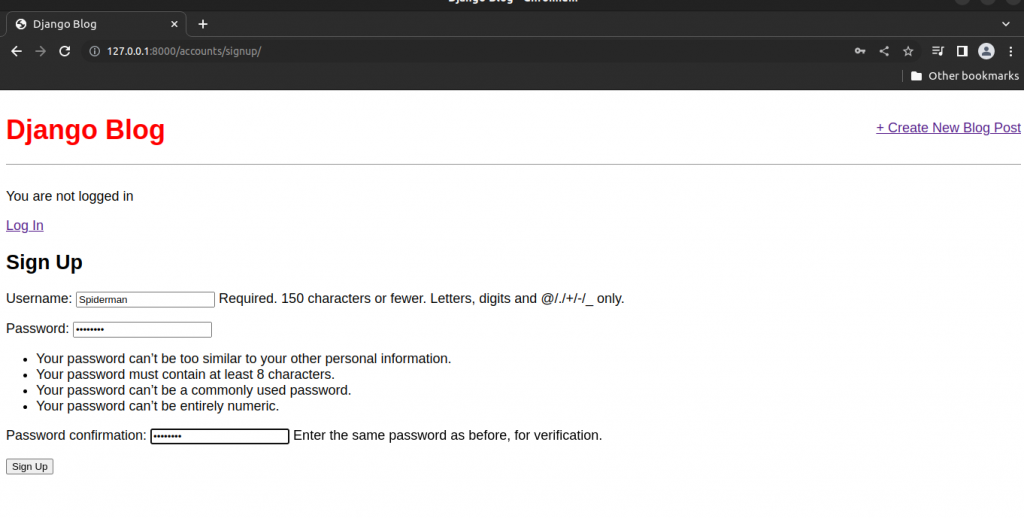
After signup, you will be redirected to login page.
Sign Up Link
We need to add a sign up link to the logged out homepage.
Edit the base.html as follows.
............................
<p>You are not logged in </p>
<a href="{% url 'login' %}">Log In</a>
<br>
<a href="{% url 'signup' %}">Sign Up</a>
.............................
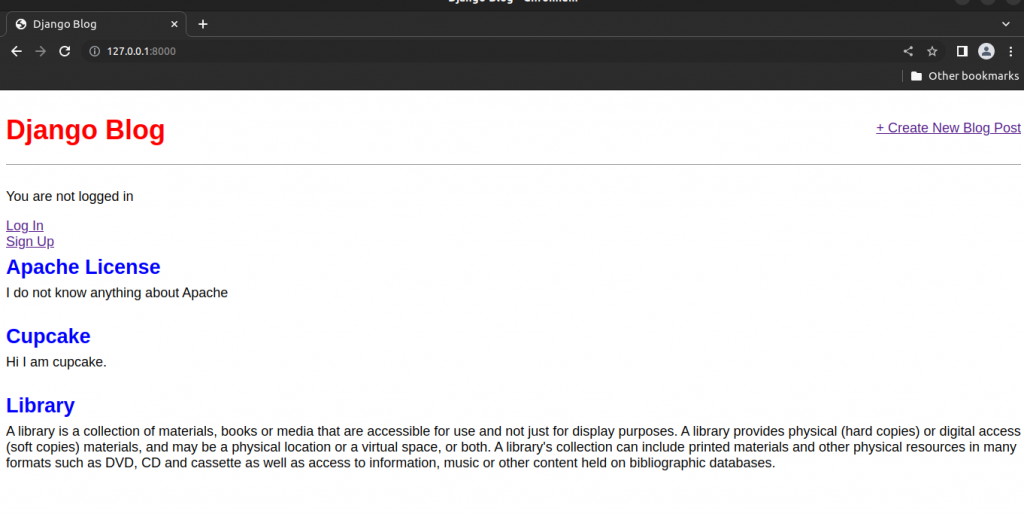